※ HTML, CSS, JavaScript 등 예제 코드 및 튜토리얼 페이지
https://www.w3schools.com/default.asp
W3Schools Free Online Web Tutorials
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
CSS를 적용시킬 요소 선택
1. 태그명(name)-- 중복가능
2. id--중복불가
3. class -- 그룹으로 같이 묶고 싶으면 같게 설정
작성법
1. 페이지 전체 적용 ==> <head> <style> 에 정의
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style type="text/css">
#div1{
width:100px;
height:100px;
background-color:#ff0000;
}
</style>
</head>
<body>
<div id="div1">aaa</div>
</body>
2. 요소 한개에 적용 ==> style 속성으로 css 작성 (우선 적용됨)
<h2 style="width:100px; height:100px;background-color:pink">fff</h2>
3. CSS 파일 만들어서 링크로 달기
▼ CSS
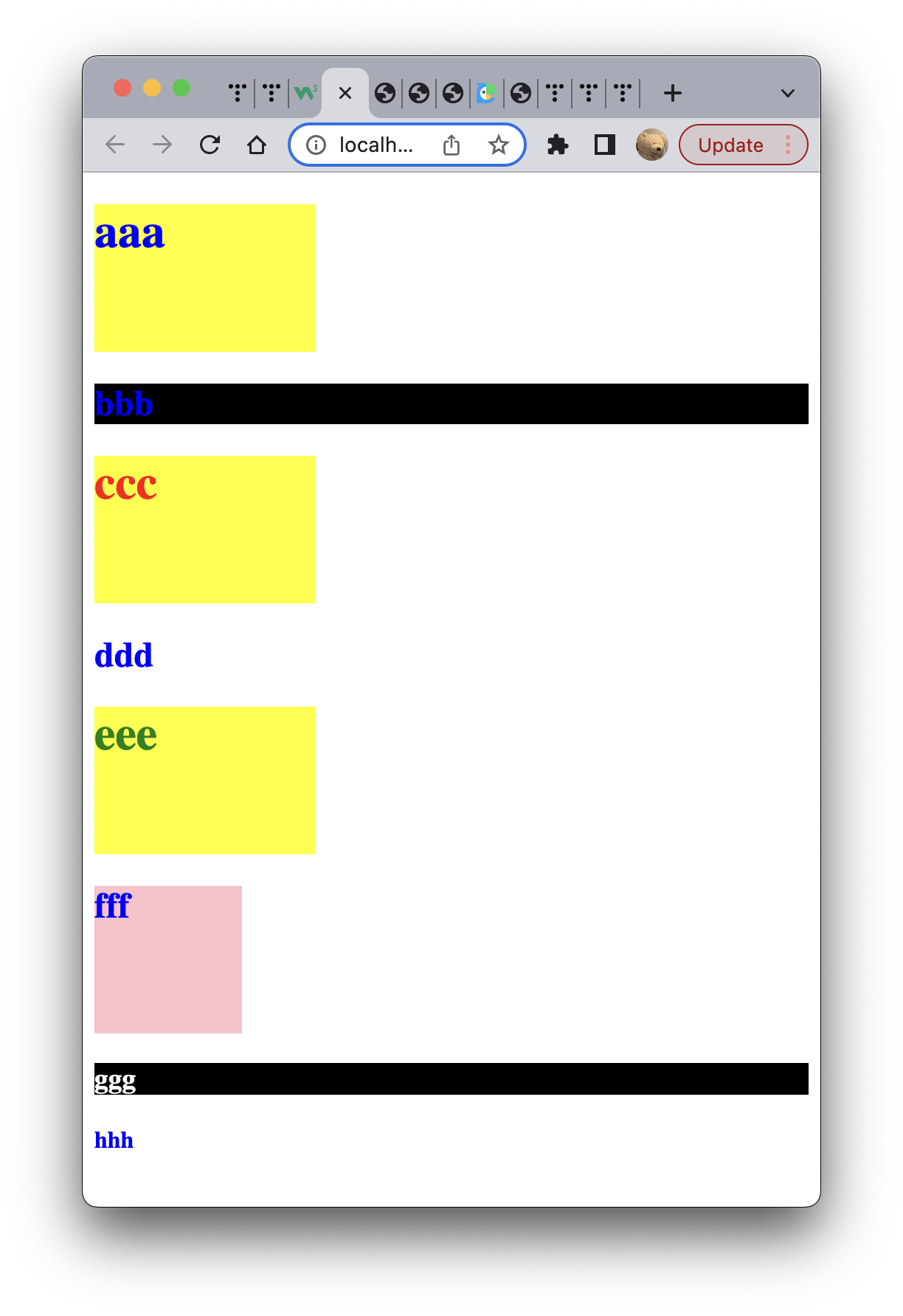
@charset "EUC-KR";
/* h1 태그 전체 css */
h1{
width:150px;
height:100px;
background-color:yellow;
}
/* body 전체 css */
body{
color: blue;
}
/* id가 h1_1인 요소 */
#h1_1{
color:red;
}
/* h1 태그 중에서 id가 h1_1인 요소 */
h1#h1_2{
color:green;
}
/* 태그 종료 상관없이 class가 c1인 요소 (.은 class) */
.c1{
background-color:black;
}
/* h3 태그 중 class가 c2인 요소 */
h3.c2{
background-color:black;
color:white;
}
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<!-- css 파일 링크 -->
<link rel="stylesheet" href="mystyle.css">
</head>
<body>
<h1>aaa</h1>
<h2 class="c1">bbb</h2>
<h1 id="h1_1">ccc</h1>
<h2>ddd</h2>
<h1 id="h1_2">eee</h1>
<h2 class="c1" style="width:100px;height:100px;background-color:pink">fff</h2>
<h3 class="c2">ggg</h3>
<h4 class="c2">hhh</h4>
</body>
</html>
포지션으로 위치 지정
- static -- 순차대로 배치 (지정 안하는 것과 같음)
- relative -- 상대 위치 (기준점(바로 앞 요소)으로부터 위, 아래, 왼, 오른쪽으로 얼만큼 떨어진 위치로 설정)
- absolute -- 절대 위치 (기준점은 왼쪽 꼭대기 -- 바뀌지 않음)
- fixed -- 고정 위치 (스크롤이 이동해도 항상 제자리)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style type="text/css">
#div1{
width:100px;
height:100px;
background-color:#ff0000;
position:static;
}
#div2{
width:100px;
height:100px;
background-color:#00ff00;
position:relative;
top:100px;
left:50px;
}
#div3{
width:100px;
height:100px;
background-color:#0000ff;
position:absolute;
top:50px;
left:50px;
}
</style>
</head>
<body>
<div id="div1">aaa</div>
<div id="div2">bbb</div>
<div id="div3">ccc</div>
</body>
</html>
버튼에 이미지 넣기
background-image:url("경로")
- 버튼 크기보다 작은 이미지를 넣으면 repeat이 되어서 패턴처럼 나옴.
backgrond-repeat: repeat-x; (가로축만 반복)
backgrond-repeat: repeat-y; (세로축만 반복)
backgrond-repeat: no-repeat; (반복 없이 하나만 띄움)
background-position: left( /center/right ) top( /bottom/left/right/center ); (이미지 위치 지정)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style type="text/css">
input[type=button]{
width:300px;
height:150px;
}
#b1{
background-color:gray;
}
#b2{
background-image:url("../img/b.png");
}
#b3{
background-image:url("../img/b.png");
background-repeat:repeat-x;
}
#b4{
background-image:url("../img/b.png");
background-repeat:repeat-y;
}
#b5{
background-image:url("../img/b.png");
background-repeat:no-repeat;
background-position:right bottom;
}
</style>
</head>
<body>
<input type="button" id="b1" value="button1"><br/>
<input type="button" id="b2" value="button2"><br/>
<input type="button" id="b3" value="button2"><br/>
<input type="button" id="b4" value="button2"><br/>
<input type="button" id="b5" value="button2"><br/>
</body>
</html>
테두리 스타일 지정 (border-style)
dotted / dashed / solid / double / groove / ridge / inset / outset / none / hidden
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style>
div{
width:100px; height:50px;
}
#d1 {border-style: dotted;}
#d2 {border-style: dashed;}
#d3 {border-style: solid;}
#d4 {border-style: double;}
#d5 {border-style: groove;}
#d6 {border-style: ridge;}
#d7 {border-style: inset;}
#d8 {border-style: outset;}
#d9 {border-style: none;}
#d10 {border-style: hidden;}
#d11 {border-style: dotted dashed solid double;}
</style>
</head>
<body>
<div id="d1"> dotted</div><br/>
<div id="d2"> dashed</div><br/>
<div id="d3"> solid</div><br/>
<div id="d4"> double</div><br/>
<div id="d5"> groove</div><br/>
<div id="d6"> ridge</div><br/>
<div id="d7"> inset</div><br/>
<div id="d8"> outset</div><br/>
<div id="d9"> none</div><br/>
<div id="d10"> hidden</div><br/>
<div id="d11"> dotted dashed solid double</div><br/><br/>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style>
div{
width:100px; height:50px;
}
.a{
border-style:solid;
border-color:red;
}
</style>
</head>
<body>
<div class="a" style="border-width:1px">1px</div><br/>
<div class="a" style="border-width:2px">2px</div><br/>
<div class="a" style="border-width:3px">3px</div><br/><br/>
<div style="border:solid 2px blue">한 번에 정의</div><br/>
</body>
</html>