https://intheham.tistory.com/48
Servlet과 JSP를 활용한 회원가입 폼
0. /Servers/server.xml 수정 - 한글이 계속 깨질 경우 한글 안 깨지게 server.xml에 추가 URIEncoding = "UTF-8" -- jsp의 pageEncoding 과 값 맞추기 (UTF-8 이나 EUC-KR 중 하나로 통일) - Servlet의 doGet()에 추가 request.setCha
intheham.tistory.com
https://intheham.tistory.com/51
(2) Servlet 과 JSP를 활용한 로그인 폼 (Member) -- DB 연결 없이
https://intheham.tistory.com/48 Servlet과 JSP를 활용한 회원가입 폼 0. /Servers/server.xml 수정 - 한글이 계속 깨질 경우 한글 안 깨지게 server.xml에 추가 URIEncoding = "UTF-8" -- jsp의 pageEncoding 과 값 맞추기 (UTF-8 이
intheham.tistory.com
------------------------
상품 등록 -- void insert(ProductVo vo)
번호로 검색 -- ProductVo selectByNum(int num)
제품명으로 검색 -- ArrayList<ProductVo> selectByName(String name)
가격으로 검색 -- ArrayList<ProductVo> selectByPrice(int price1, int price2)
전체목록 -- ArrayList<ProductVo> selectAll()
가격, 수량 수정 -- void update(ProductVo vo)
삭제 -- void delete(int num)
-------------------------
1. 홈화면 ( index.jsp ) ---> 2. 상품 전체보기
- "상품목록" 링크를 누르면 List.java 로 이동
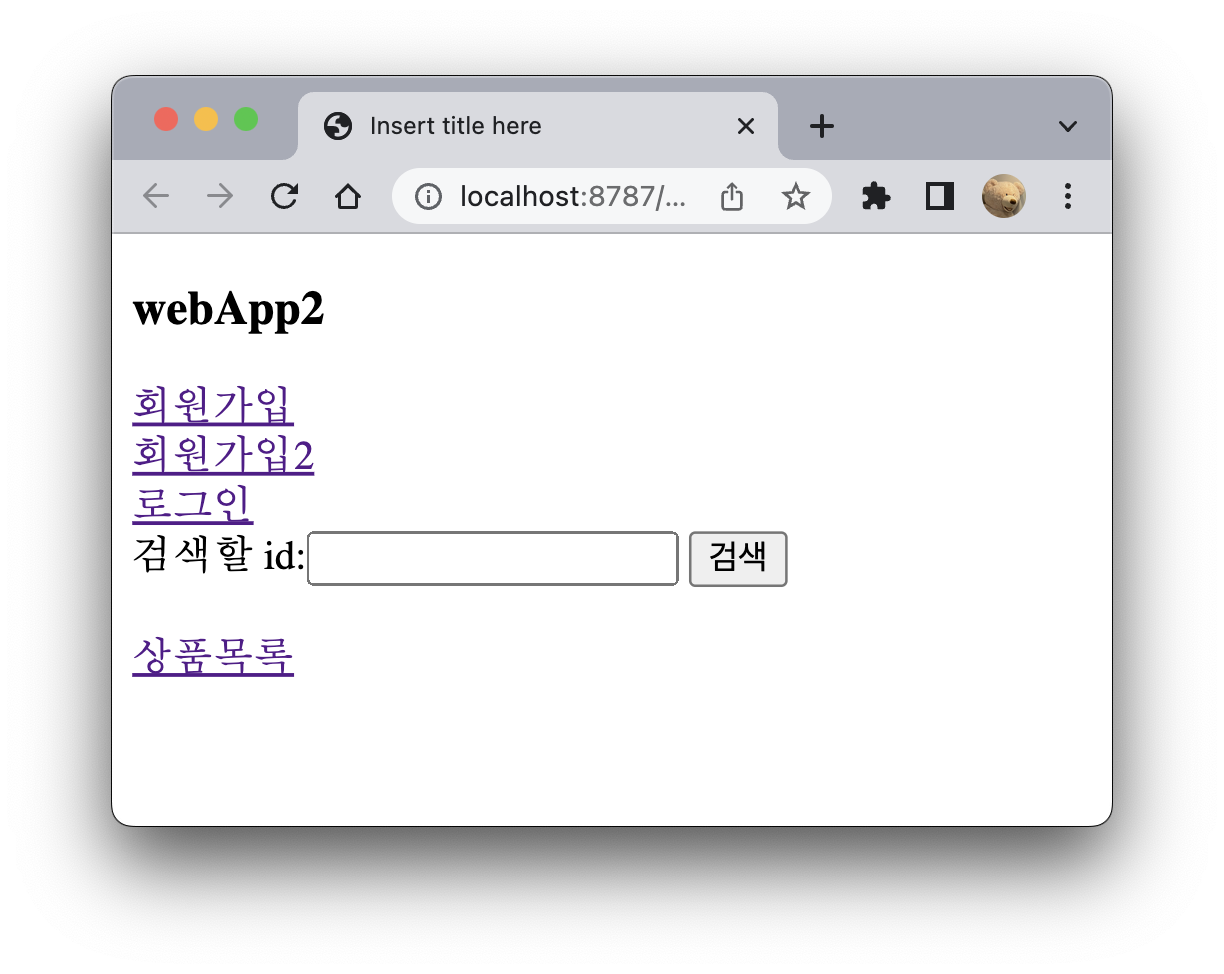
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>webApp2</h3>
<a href="/webApp2/Join">회원가입</a><br/>
<a href="/webApp2/test/Join">회원가입2</a><br/>
<a href = "/webApp2/test/Login">로그인</a><br/>
<form action="/webApp2/MyInfo" method="post">
검색할 id:<input type="text" name="id">
<input type="submit" value = "검색">
</form><br/>
<!-- 1. 상품 전체 목록 -->
<a href="/webApp2/product/list">상품목록</a><br/>
</body>
</html>
2. 상품 전체보기 ( List.java ) -- 3. 상품 추가
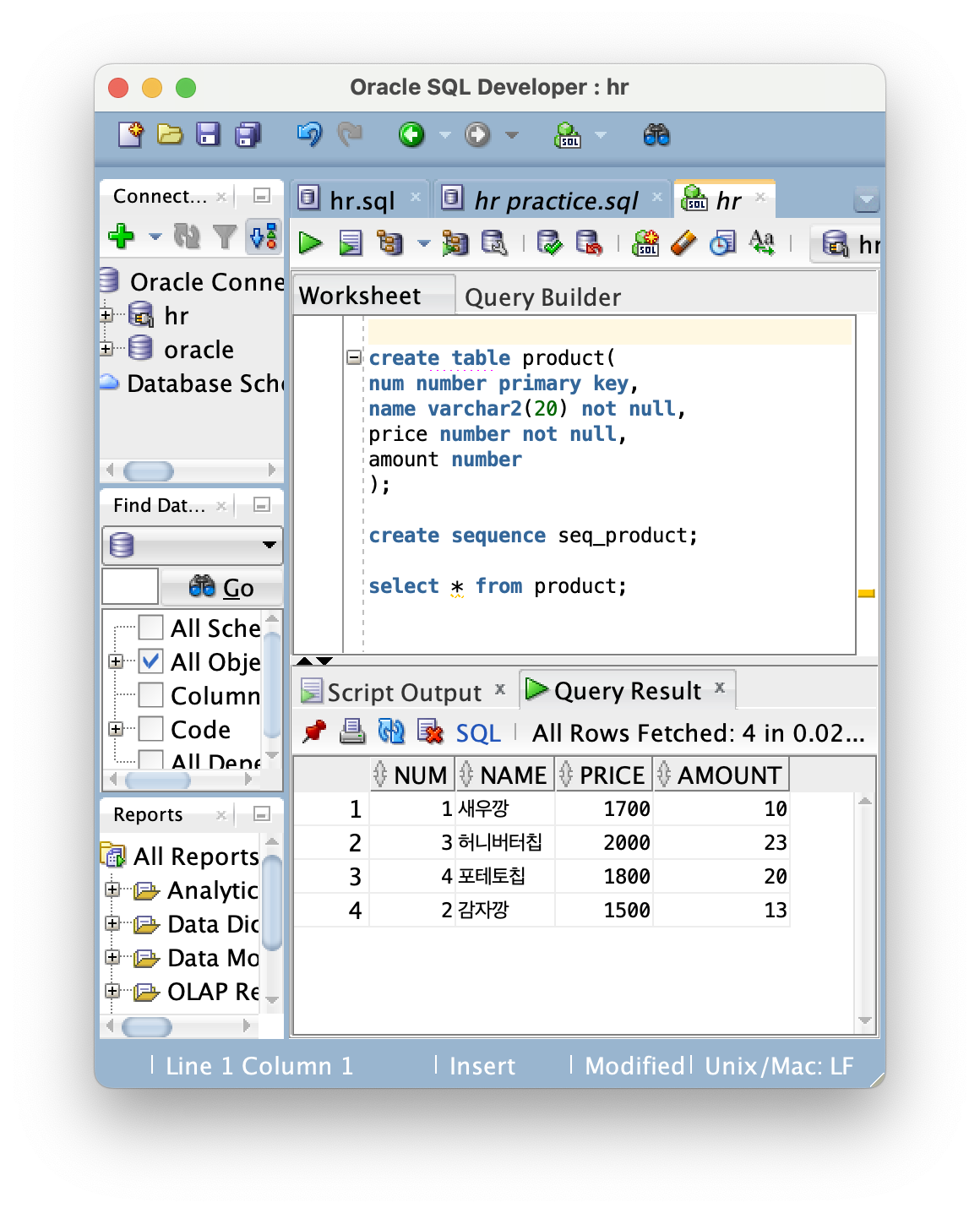
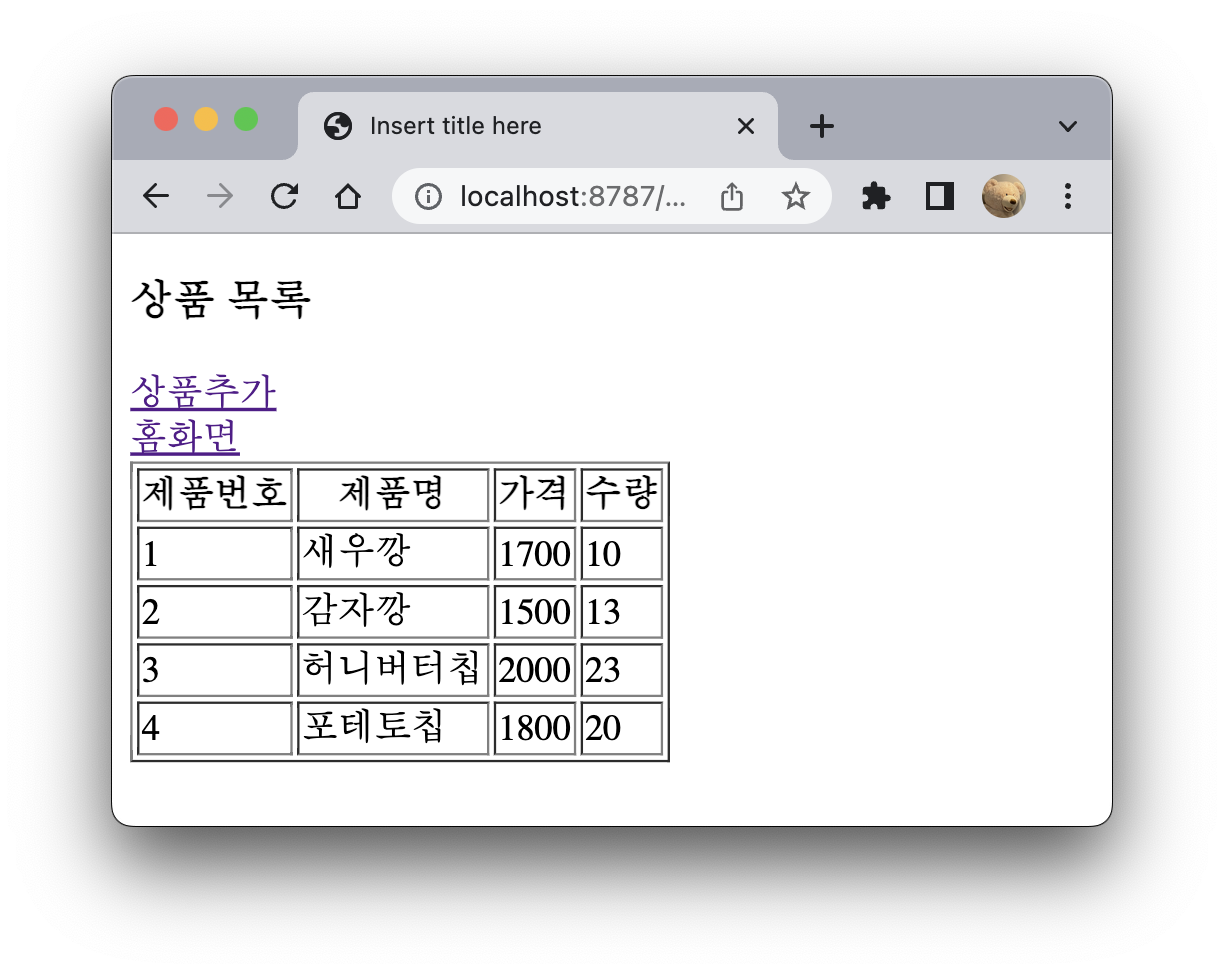
(1) doGet() -- 상품 리스트를 Service에서 전체 검색 후 그 결과를 list.jsp에 보냄
(2) list.jsp -- 상품 리스트를 표에 담아 보여줌
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
- jsp에서 자바코드 역할을 하는 라이브러리를 쓸 수 있게 해줌. (최상단에 코드 붙여넣기)
<c:forEach var="변수명" items="${배열 or 리스트 }"></c:forEach>
- for문 기능 구현
- 자동으로 배열/리스트 길이만큼 루프 돌며 "변수명"에 값을 넣어줌.
(2-1) 상품추가 링크를 누르면 Add.java 로 감 ------ 3. 상품 추가
(2-2) 홈화면 링크 누르면 index.jsp 로 감
▼ List (servlet) -- (1)
@WebServlet("/product/list")
public class List extends HttpServlet {
private static final long serialVersionUID = 1L;
public List() {
super();
}
// 전체 목록 보여주기는 1 단계만 필요함.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ProductService service = new ProductService();
ArrayList<ProductVo> list = service.getAll(); // 전체검색
request.setAttribute("list", list); // request에 결과 담기
// 뷰페이지에 보여주기
RequestDispatcher dis = request.getRequestDispatcher("/product/list.jsp");
dis.forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
▼ list.jsp -- (2)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%> <!-- page: jsp 설정 지시자 -->
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!-- 자바코드를 쓰면 너무 지저분하니까 라이브러리로 만들어놓음! -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 목록</h3>
<!-- 3. 상품 추가 -->
<a href="/webApp2/product/add">상품추가</a><br/>
<!-- 홈화면 돌아가기 -->
<a href="/webApp2/index.jsp">홈화면</a><br/>
<table border="1">
<tr><th>제품번호</th><th>제품명</th><th>가격</th><th>수량</th></tr>
<!-- JSTL 의 for문 역할의 라이브러리 -->
<c:forEach var="vo" items="${list }">
<tr><td>${vo.num}</td><td>${vo.name}</td><td>${vo.price}</td><td>${vo.amount}</td>
</c:forEach>
</table>
</form>
</body>
</html>
3. 상품 추가 (Add.java)
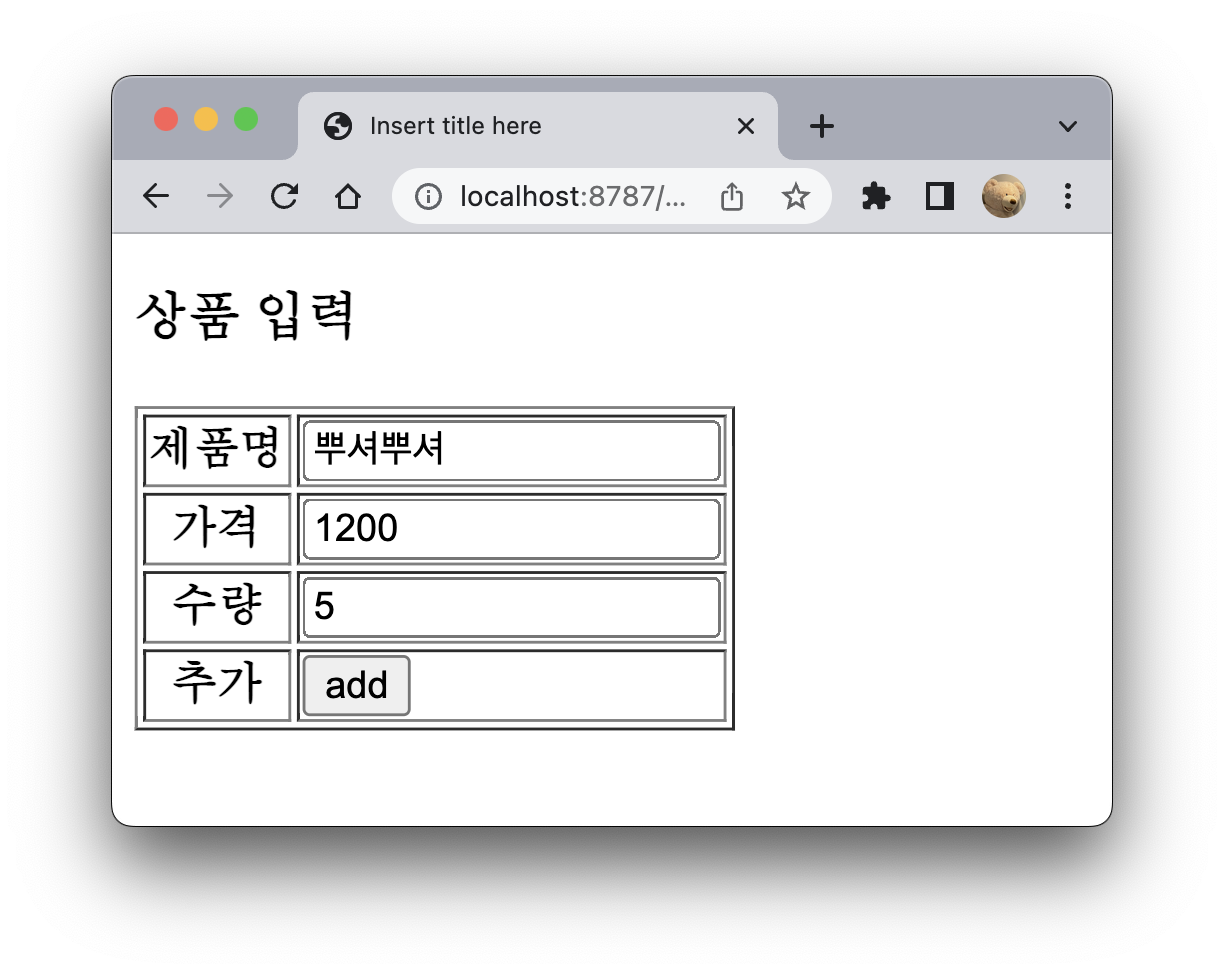
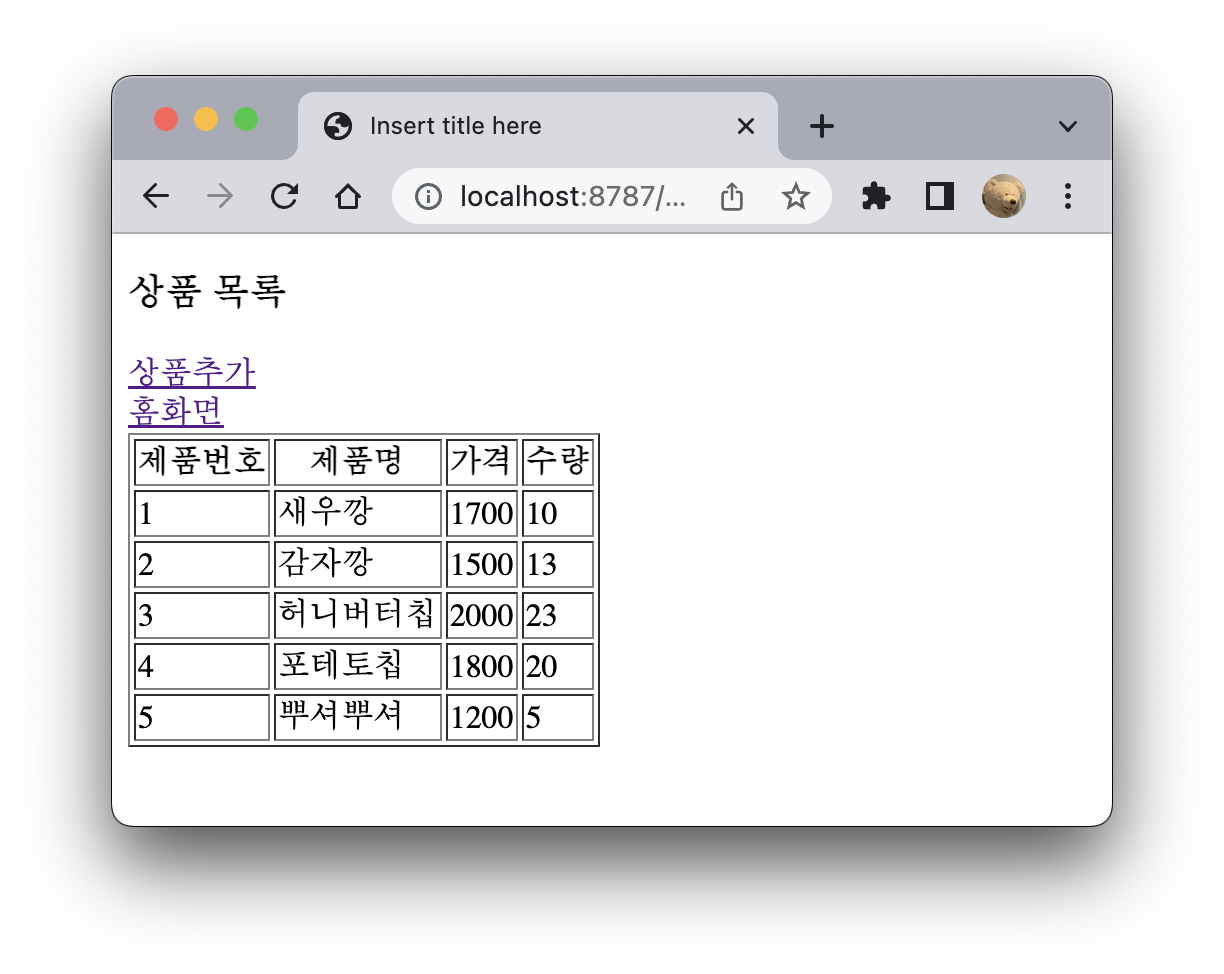
(1) doGet() -- 상품 입력 폼으로 넘어감 (add.jsp)
(2) add.jsp -- 사용자가 폼에 값을 입력하고 submit 하면 그 값을 가지고 Add.java로 넘어감
(3) doPost() -- 입력한 값을 읽어와서 Service의 메소드를 통해 db에 저장 -> 전체 리스트 불러오기 (List.java)
Integer.parseInt()
-- int로 형변환
-- request.getParameter 는 무조건 값을 String 으로 반환.
-- 반환값을 int 변수에 넣으려면 형변환 필요함.
String name = request.getParameter("name");
int price = Integer.parseInt(request.getParameter("price"));
▼ Add (servlet) -- (1), (3)
@WebServlet("/product/add")
public class Add extends HttpServlet {
private static final long serialVersionUID = 1L;
public Add() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 상품 추가 폼
RequestDispatcher dis = request.getRequestDispatcher("/product/add.jsp");
dis.forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 한글 안깨지게!
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
// 폼파라메터에 입력된 상품정보 읽어와서 dq에 저장
String name = request.getParameter("name");
int price = Integer.parseInt(request.getParameter("price"));
int amount = Integer.parseInt(request.getParameter("amount"));
ProductService service = new ProductService();
service.addProduct(new ProductVo(0, name, price, amount));
// 완료 후 List.java로 가기 -- 전체 목록 리프레시
// redirect를 써서 링크 타고 들어가면 get 방식으로 doGet() 호출
response.sendRedirect("/webApp2/product/list");
}
}
▼ add.jsp -- (2)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 입력</h3>
<form action = "/webApp2/product/add" method = "post">
<table border = "1">
<tr><th>제품명</th><td><input type = "text" name = "name"></td></tr>
<tr><th>가격</th><td><input type = "number" name = "price"></td></tr>
<tr><th>수량</th><td><input type = "number" name = "amount"></td></tr>
<tr><th>추가</th><td><input type = "submit" value = "add"></td></tr>
</table>
</form>
</body>
</html>
0. 총코드
ProductVo
package product;
public class ProductVo {
private int num;
private String name;
private int price;
private int amount;
public ProductVo() {}
public ProductVo(int num, String name, int price, int amount) {
super();
this.num = num;
this.name = name;
this.price = price;
this.amount = amount;
}
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public int getAmount() {
return amount;
}
public void setAmount(int amount) {
this.amount = amount;
}
@Override
public String toString() {
return "ProductVo [num=" + num + ", name=" + name + ", price=" + price + ", amount=" + amount + "]";
}
}
ProductDao
package product;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import conn.DBConnect;
public class ProductDao {
private DBConnect dbconn;
public ProductDao() {
dbconn = DBConnect.getInstance();
}
// 상품 등록 -- void insert(ProductVo vo)
public void insert(ProductVo vo) {
Connection conn = dbconn.conn();
String sql = "insert into product values(seq_product.nextval, ?, ?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, vo.getName());
pstmt.setInt(2, vo.getPrice());
pstmt.setInt(3, vo.getAmount());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 추가되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 번호로 검색 -- ProductVo selectByNum(int num)
public ProductVo selectByNum(int num) {
ArrayList<ProductVo> list = new ArrayList<>();
ProductVo vo = null;
Connection conn = dbconn.conn();
String sql = "select * from product where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
if (rs.next()) { // 검색결과가 있으면 실행
return new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return null; // 검색된거 없으면 널값 리턴
}
// 제품명으로 검색 -- ArrayList<ProductVo> selectByName(String name)
public ArrayList<ProductVo> selectByName(String name) {
ArrayList<ProductVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from product where name = ? order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, name);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
list.add(new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 가격으로 검색 -- ArrayList<ProductVo> selectByPrice(int price1, int price2)
public ArrayList<ProductVo> selectByPrice(int price1, int price2) {
ArrayList<ProductVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from product where price between ? and ? order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, price1);
pstmt.setInt(2, price2);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
list.add(new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 전체목록 -- ArrayList<ProductVo> selectAll()
public ArrayList<ProductVo> selectAll() {
ArrayList<ProductVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from product order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
list.add(new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 가격, 수량 수정 -- void update(ProductVo vo)
public void update(ProductVo vo) {
Connection conn = dbconn.conn();
String sql = "update product set price = ?, amount = ? where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, vo.getPrice());
pstmt.setInt(2, vo.getAmount());
pstmt.setInt(3, vo.getNum());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 수정되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 삭제 -- void delete(int num)
public void delete(int num) {
Connection conn = dbconn.conn();
String sql = "delete product where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
int number = pstmt.executeUpdate();
System.out.println(number + "줄이 삭제되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
ProductService
package product;
import java.util.ArrayList;
public class ProductService {
private ProductDao dao;
public ProductService() {
dao = new ProductDao();
}
// 제품 추가
public void addProduct(ProductVo vo) {
dao.insert(vo);
}
// 번호로 검색
public ProductVo getByNum(int num) {
return dao.selectByNum(num);
}
// 제품명으로 검색
public ArrayList<ProductVo> getByName(String name) {
return dao.selectByName(name);
}
// 가격으로 검색
public ArrayList<ProductVo> getByPrice(int p1, int p2) {
return dao.selectByPrice(p1, p2);
}
// 전체 검색
public ArrayList<ProductVo> getAll() {
return dao.selectAll();
}
// 가격, 수량 수정
public void editProduct(ProductVo vo) {
dao.update(vo);
}
// 삭제
public void delProduct(int num) {
dao.delete(num);
}
}
Add.java
package product.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import product.ProductService;
import product.ProductVo;
/**
* Servlet implementation class Add
*/
@WebServlet("/product/add")
public class Add extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Add() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
// 상품 추가 폼
RequestDispatcher dis = request.getRequestDispatcher("/product/add.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
// 한글 안깨지게!
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
// 폼파라메터에 입력된 상품정보 읽어와서 dq에 저장
// request.getParameter 는 무조건 값을 String 으로 반환.
// Integer.parseInt() : int로 형변환
String name = request.getParameter("name");
int price = Integer.parseInt(request.getParameter("price"));
int amount = Integer.parseInt(request.getParameter("amount"));
ProductService service = new ProductService();
service.addProduct(new ProductVo(0, name, price, amount));
// 완료 후 List.java로 가기 -- 전체 목록 리프레시
// redirect를 써서 링크 타고 들어가면 get 방식으로 doGet() 호출
response.sendRedirect("/webApp2/product/list");
}
}
List.java
package product.controller;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import product.ProductService;
import product.ProductVo;
/**
* Servlet implementation class All
*/
@WebServlet("/product/list")
public class List extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public List() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
// 전체 목록 보여주기는 1 단계만 필요함.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
ProductService service = new ProductService();
ArrayList<ProductVo> list = service.getAll(); // 전체검색
request.setAttribute("list", list); // request에 결과 담기
// 뷰페이지에 보여주기
RequestDispatcher dis = request.getRequestDispatcher("/product/list.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>webApp2</h3>
<a href="/webApp2/Join">회원가입</a><br/>
<!-- 5. 회원가입2 -->
<a href="/webApp2/test/Join">회원가입2</a><br/>
<!-- 4. 로그인 -- /test/Login에 get방식 요청 -->
<a href = "/webApp2/test/Login">로그인</a><br/>
<!-- 3. id 검색 -->
<form action="/webApp2/MyInfo" method="post">
검색할 id:<input type="text" name="id">
<input type="submit" value = "검색">
</form><br/>
<!-- 7. 상품 전체 목록 -->
<a href="/webApp2/product/list">상품목록</a><br/>
</body>
</html>
add.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 입력</h3>
<form action = "/webApp2/product/add" method = "post">
<table border = "1">
<tr><th>제품명</th><td><input type = "text" name = "name"></td></tr>
<tr><th>가격</th><td><input type = "number" name = "price"></td></tr>
<tr><th>수량</th><td><input type = "number" name = "amount"></td></tr>
<tr><th>추가</th><td><input type = "submit" value = "add"></td></tr>
</table>
</form>
</body>
</html>
list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%> <!-- page: jsp 설정 지시자 -->
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!-- 자바코드를 쓰면 너무 지저분하니까 라이브러리로 만들어놓음! -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 목록</h3>
<!-- 6. 상품 추가 -->
<a href="/webApp2/product/add">상품추가</a><br/>
<!-- 홈화면 돌아가기 -->
<a href="/webApp2/index.jsp">홈화면</a><br/>
<table border="1">
<tr><th>제품번호</th><th>제품명</th><th>가격</th><th>수량</th></tr>
<!-- for문 - 자동으로 list.size 만큼 루프돎. 자동으로 "vo"에 넣어줌. -->
<!-- items = "리스트 or 배열" -->
<!-- ProductVo vo = list.get(i) 와 같음. -->
<c:forEach var="vo" items="${list }">
<tr><td>${vo.num}</td><td>${vo.name}</td><td>${vo.price}</td><td>${vo.amount}</td>
</c:forEach>
</table>
</form>
</body>
</html>
https://intheham.tistory.com/48
Servlet과 JSP를 활용한 회원가입 폼
0. /Servers/server.xml 수정 - 한글이 계속 깨질 경우 한글 안 깨지게 server.xml에 추가 URIEncoding = "UTF-8" -- jsp의 pageEncoding 과 값 맞추기 (UTF-8 이나 EUC-KR 중 하나로 통일) - Servlet의 doGet()에 추가 request.setCha
intheham.tistory.com
https://intheham.tistory.com/51
(2) Servlet 과 JSP를 활용한 로그인 폼 (Member) -- DB 연결 없이
https://intheham.tistory.com/48 Servlet과 JSP를 활용한 회원가입 폼 0. /Servers/server.xml 수정 - 한글이 계속 깨질 경우 한글 안 깨지게 server.xml에 추가 URIEncoding = "UTF-8" -- jsp의 pageEncoding 과 값 맞추기 (UTF-8 이
intheham.tistory.com
------------------------
상품 등록 -- void insert(ProductVo vo)
번호로 검색 -- ProductVo selectByNum(int num)
제품명으로 검색 -- ArrayList<ProductVo> selectByName(String name)
가격으로 검색 -- ArrayList<ProductVo> selectByPrice(int price1, int price2)
전체목록 -- ArrayList<ProductVo> selectAll()
가격, 수량 수정 -- void update(ProductVo vo)
삭제 -- void delete(int num)
-------------------------
1. 홈화면 ( index.jsp ) ---> 2. 상품 전체보기
- "상품목록" 링크를 누르면 List.java 로 이동
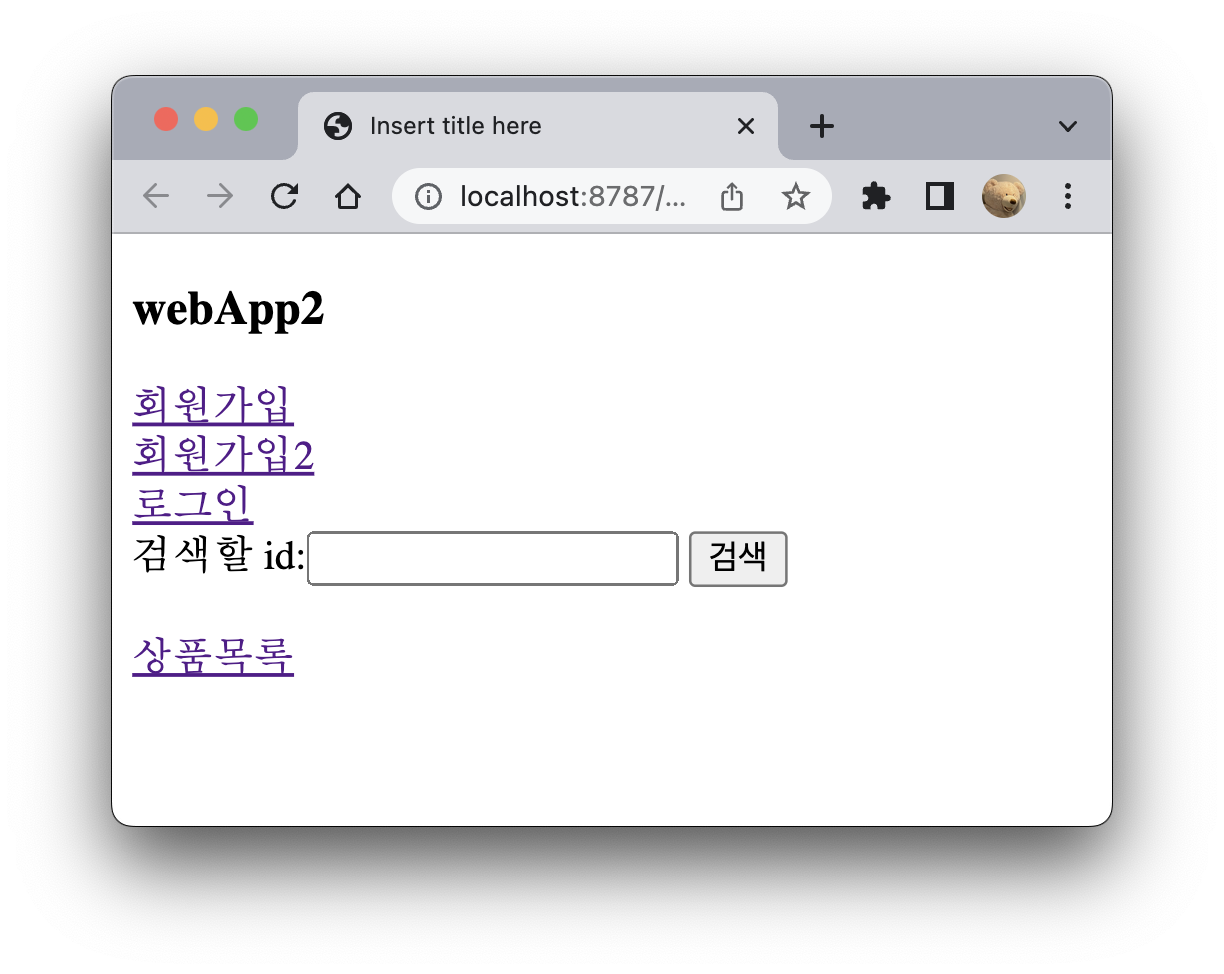
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>webApp2</h3>
<a href="/webApp2/Join">회원가입</a><br/>
<a href="/webApp2/test/Join">회원가입2</a><br/>
<a href = "/webApp2/test/Login">로그인</a><br/>
<form action="/webApp2/MyInfo" method="post">
검색할 id:<input type="text" name="id">
<input type="submit" value = "검색">
</form><br/>
<!-- 1. 상품 전체 목록 -->
<a href="/webApp2/product/list">상품목록</a><br/>
</body>
</html>
2. 상품 전체보기 ( List.java ) -- 3. 상품 추가
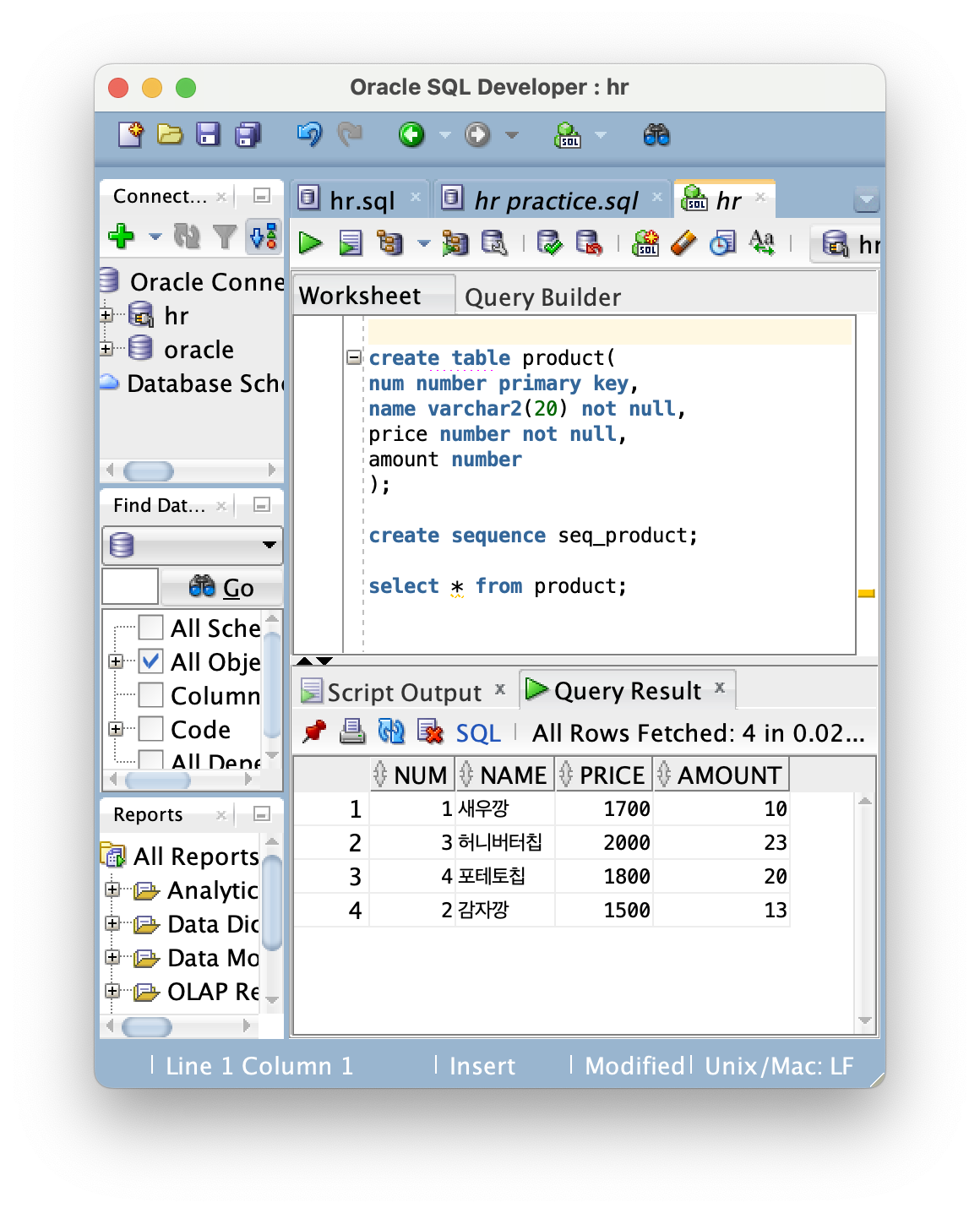
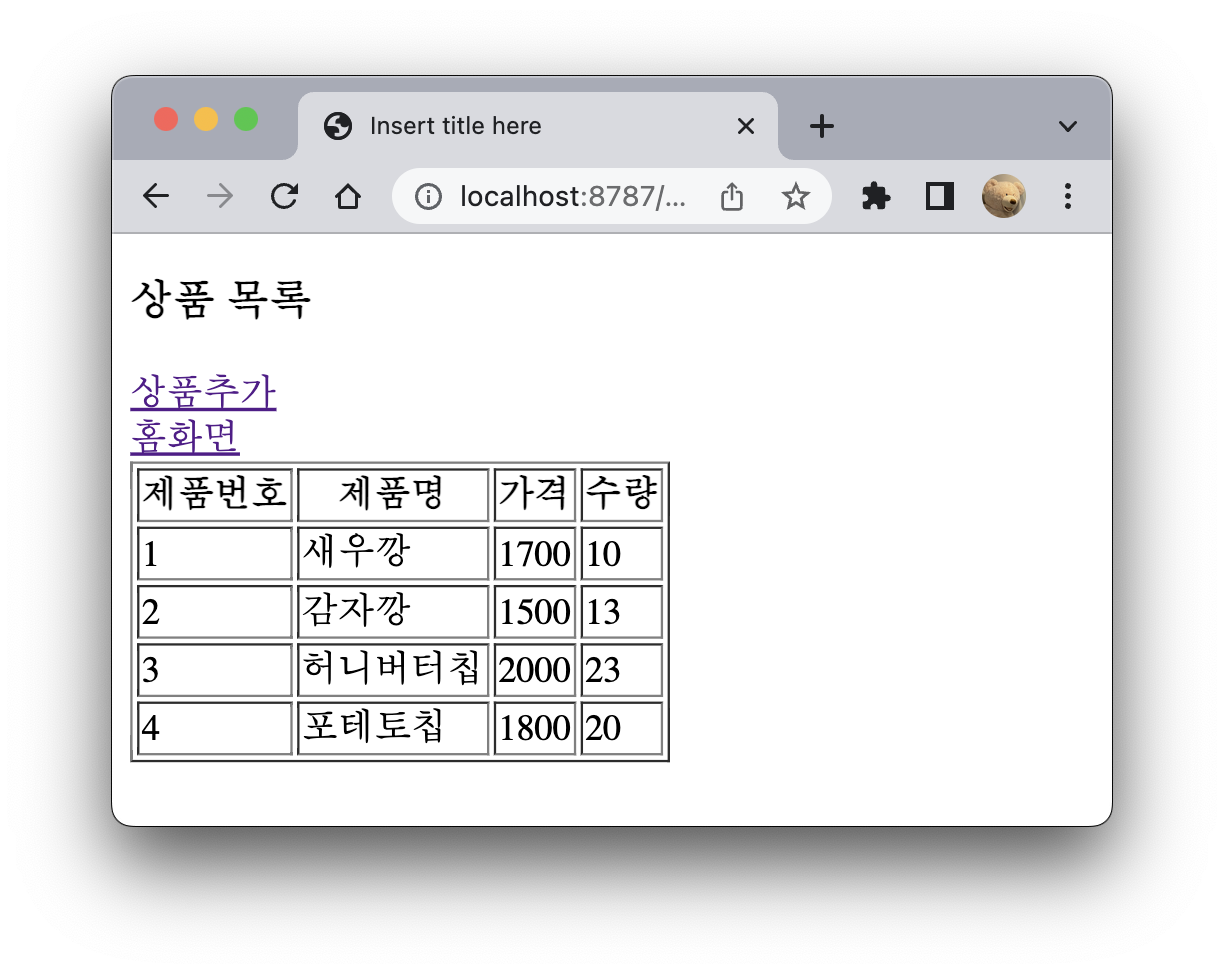
(1) doGet() -- 상품 리스트를 Service에서 전체 검색 후 그 결과를 list.jsp에 보냄
(2) list.jsp -- 상품 리스트를 표에 담아 보여줌
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
- jsp에서 자바코드 역할을 하는 라이브러리를 쓸 수 있게 해줌. (최상단에 코드 붙여넣기)
<c:forEach var="변수명" items="${배열 or 리스트 }"></c:forEach>
- for문 기능 구현
- 자동으로 배열/리스트 길이만큼 루프 돌며 "변수명"에 값을 넣어줌.
(2-1) 상품추가 링크를 누르면 Add.java 로 감 ------ 3. 상품 추가
(2-2) 홈화면 링크 누르면 index.jsp 로 감
▼ List (servlet) -- (1)
@WebServlet("/product/list")
public class List extends HttpServlet {
private static final long serialVersionUID = 1L;
public List() {
super();
}
// 전체 목록 보여주기는 1 단계만 필요함.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ProductService service = new ProductService();
ArrayList<ProductVo> list = service.getAll(); // 전체검색
request.setAttribute("list", list); // request에 결과 담기
// 뷰페이지에 보여주기
RequestDispatcher dis = request.getRequestDispatcher("/product/list.jsp");
dis.forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
▼ list.jsp -- (2)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%> <!-- page: jsp 설정 지시자 -->
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!-- 자바코드를 쓰면 너무 지저분하니까 라이브러리로 만들어놓음! -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 목록</h3>
<!-- 3. 상품 추가 -->
<a href="/webApp2/product/add">상품추가</a><br/>
<!-- 홈화면 돌아가기 -->
<a href="/webApp2/index.jsp">홈화면</a><br/>
<table border="1">
<tr><th>제품번호</th><th>제품명</th><th>가격</th><th>수량</th></tr>
<!-- JSTL 의 for문 역할의 라이브러리 -->
<c:forEach var="vo" items="${list }">
<tr><td>${vo.num}</td><td>${vo.name}</td><td>${vo.price}</td><td>${vo.amount}</td>
</c:forEach>
</table>
</form>
</body>
</html>
3. 상품 추가 (Add.java)
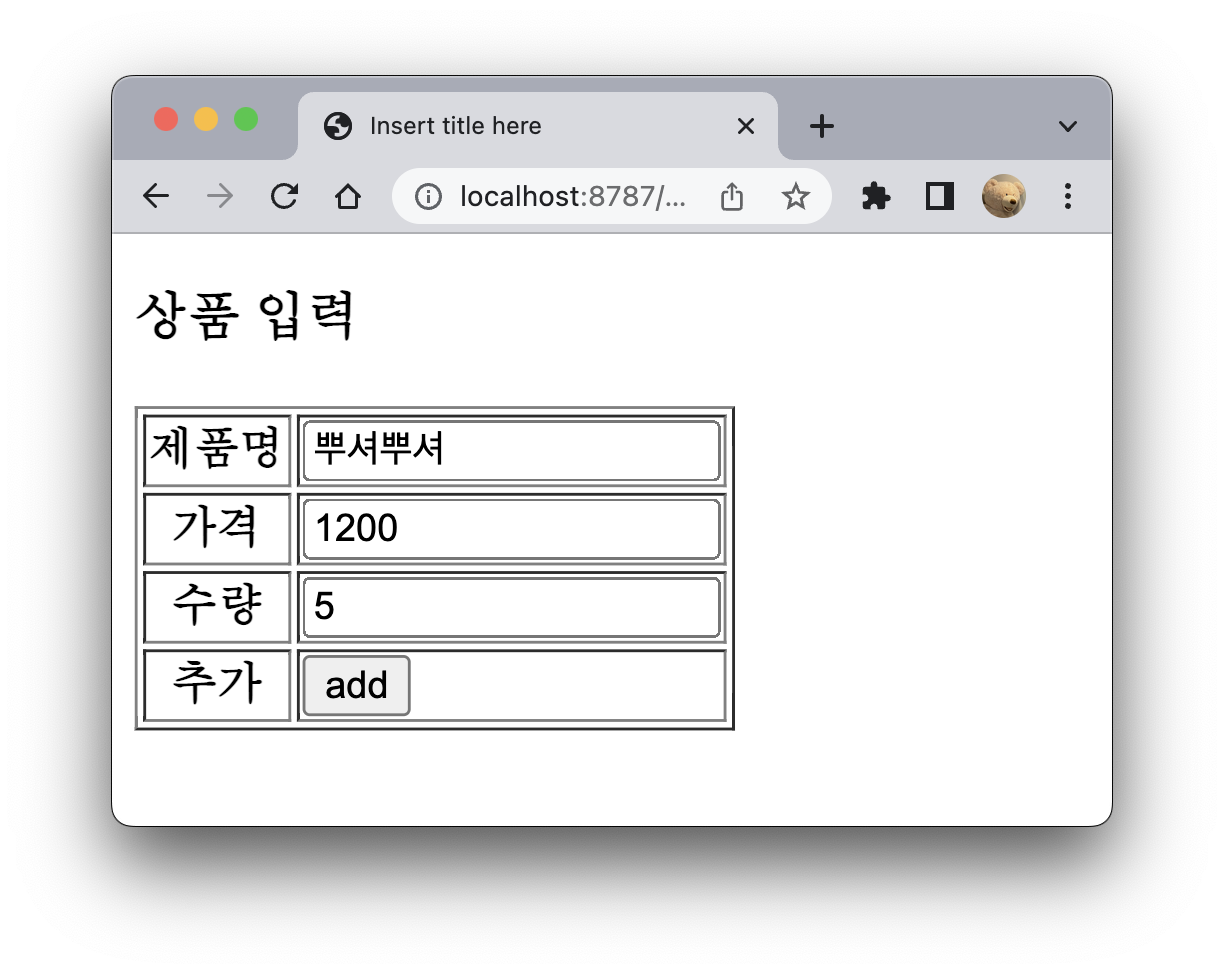
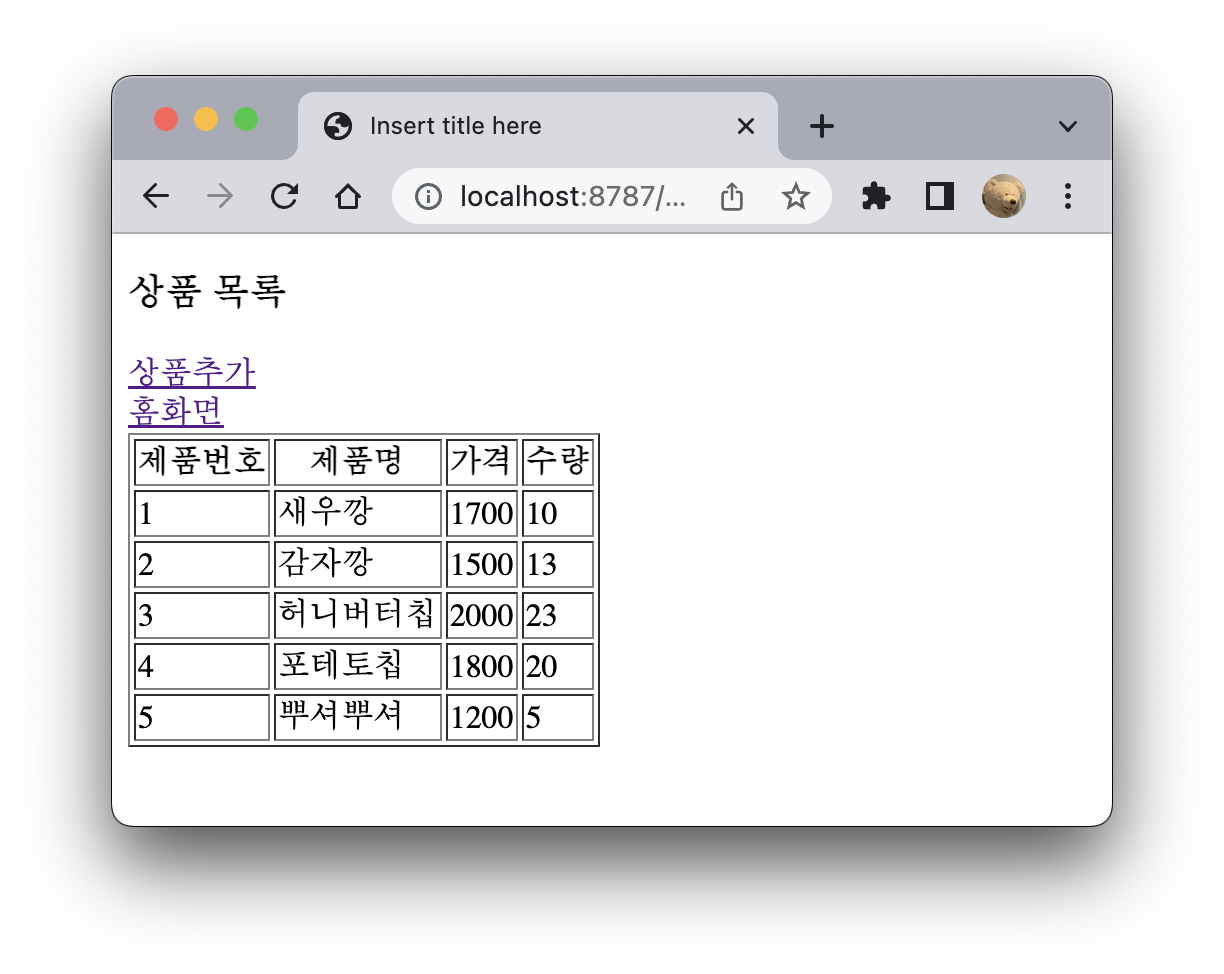
(1) doGet() -- 상품 입력 폼으로 넘어감 (add.jsp)
(2) add.jsp -- 사용자가 폼에 값을 입력하고 submit 하면 그 값을 가지고 Add.java로 넘어감
(3) doPost() -- 입력한 값을 읽어와서 Service의 메소드를 통해 db에 저장 -> 전체 리스트 불러오기 (List.java)
Integer.parseInt()
-- int로 형변환
-- request.getParameter 는 무조건 값을 String 으로 반환.
-- 반환값을 int 변수에 넣으려면 형변환 필요함.
String name = request.getParameter("name");
int price = Integer.parseInt(request.getParameter("price"));
▼ Add (servlet) -- (1), (3)
@WebServlet("/product/add")
public class Add extends HttpServlet {
private static final long serialVersionUID = 1L;
public Add() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 상품 추가 폼
RequestDispatcher dis = request.getRequestDispatcher("/product/add.jsp");
dis.forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 한글 안깨지게!
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
// 폼파라메터에 입력된 상품정보 읽어와서 dq에 저장
String name = request.getParameter("name");
int price = Integer.parseInt(request.getParameter("price"));
int amount = Integer.parseInt(request.getParameter("amount"));
ProductService service = new ProductService();
service.addProduct(new ProductVo(0, name, price, amount));
// 완료 후 List.java로 가기 -- 전체 목록 리프레시
// redirect를 써서 링크 타고 들어가면 get 방식으로 doGet() 호출
response.sendRedirect("/webApp2/product/list");
}
}
▼ add.jsp -- (2)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 입력</h3>
<form action = "/webApp2/product/add" method = "post">
<table border = "1">
<tr><th>제품명</th><td><input type = "text" name = "name"></td></tr>
<tr><th>가격</th><td><input type = "number" name = "price"></td></tr>
<tr><th>수량</th><td><input type = "number" name = "amount"></td></tr>
<tr><th>추가</th><td><input type = "submit" value = "add"></td></tr>
</table>
</form>
</body>
</html>
0. 총코드
ProductVo
package product;
public class ProductVo {
private int num;
private String name;
private int price;
private int amount;
public ProductVo() {}
public ProductVo(int num, String name, int price, int amount) {
super();
this.num = num;
this.name = name;
this.price = price;
this.amount = amount;
}
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public int getAmount() {
return amount;
}
public void setAmount(int amount) {
this.amount = amount;
}
@Override
public String toString() {
return "ProductVo [num=" + num + ", name=" + name + ", price=" + price + ", amount=" + amount + "]";
}
}
ProductDao
package product;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import conn.DBConnect;
public class ProductDao {
private DBConnect dbconn;
public ProductDao() {
dbconn = DBConnect.getInstance();
}
// 상품 등록 -- void insert(ProductVo vo)
public void insert(ProductVo vo) {
Connection conn = dbconn.conn();
String sql = "insert into product values(seq_product.nextval, ?, ?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, vo.getName());
pstmt.setInt(2, vo.getPrice());
pstmt.setInt(3, vo.getAmount());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 추가되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 번호로 검색 -- ProductVo selectByNum(int num)
public ProductVo selectByNum(int num) {
ArrayList<ProductVo> list = new ArrayList<>();
ProductVo vo = null;
Connection conn = dbconn.conn();
String sql = "select * from product where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
if (rs.next()) { // 검색결과가 있으면 실행
return new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return null; // 검색된거 없으면 널값 리턴
}
// 제품명으로 검색 -- ArrayList<ProductVo> selectByName(String name)
public ArrayList<ProductVo> selectByName(String name) {
ArrayList<ProductVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from product where name = ? order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, name);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
list.add(new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 가격으로 검색 -- ArrayList<ProductVo> selectByPrice(int price1, int price2)
public ArrayList<ProductVo> selectByPrice(int price1, int price2) {
ArrayList<ProductVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from product where price between ? and ? order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, price1);
pstmt.setInt(2, price2);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
list.add(new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 전체목록 -- ArrayList<ProductVo> selectAll()
public ArrayList<ProductVo> selectAll() {
ArrayList<ProductVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from product order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
list.add(new ProductVo(rs.getInt(1), rs.getString(2), rs.getInt(3), rs.getInt(4)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 가격, 수량 수정 -- void update(ProductVo vo)
public void update(ProductVo vo) {
Connection conn = dbconn.conn();
String sql = "update product set price = ?, amount = ? where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, vo.getPrice());
pstmt.setInt(2, vo.getAmount());
pstmt.setInt(3, vo.getNum());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 수정되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 삭제 -- void delete(int num)
public void delete(int num) {
Connection conn = dbconn.conn();
String sql = "delete product where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
int number = pstmt.executeUpdate();
System.out.println(number + "줄이 삭제되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
ProductService
package product;
import java.util.ArrayList;
public class ProductService {
private ProductDao dao;
public ProductService() {
dao = new ProductDao();
}
// 제품 추가
public void addProduct(ProductVo vo) {
dao.insert(vo);
}
// 번호로 검색
public ProductVo getByNum(int num) {
return dao.selectByNum(num);
}
// 제품명으로 검색
public ArrayList<ProductVo> getByName(String name) {
return dao.selectByName(name);
}
// 가격으로 검색
public ArrayList<ProductVo> getByPrice(int p1, int p2) {
return dao.selectByPrice(p1, p2);
}
// 전체 검색
public ArrayList<ProductVo> getAll() {
return dao.selectAll();
}
// 가격, 수량 수정
public void editProduct(ProductVo vo) {
dao.update(vo);
}
// 삭제
public void delProduct(int num) {
dao.delete(num);
}
}
Add.java
package product.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import product.ProductService;
import product.ProductVo;
/**
* Servlet implementation class Add
*/
@WebServlet("/product/add")
public class Add extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Add() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
// 상품 추가 폼
RequestDispatcher dis = request.getRequestDispatcher("/product/add.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
// 한글 안깨지게!
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
// 폼파라메터에 입력된 상품정보 읽어와서 dq에 저장
// request.getParameter 는 무조건 값을 String 으로 반환.
// Integer.parseInt() : int로 형변환
String name = request.getParameter("name");
int price = Integer.parseInt(request.getParameter("price"));
int amount = Integer.parseInt(request.getParameter("amount"));
ProductService service = new ProductService();
service.addProduct(new ProductVo(0, name, price, amount));
// 완료 후 List.java로 가기 -- 전체 목록 리프레시
// redirect를 써서 링크 타고 들어가면 get 방식으로 doGet() 호출
response.sendRedirect("/webApp2/product/list");
}
}
List.java
package product.controller;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import product.ProductService;
import product.ProductVo;
/**
* Servlet implementation class All
*/
@WebServlet("/product/list")
public class List extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public List() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
// 전체 목록 보여주기는 1 단계만 필요함.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
ProductService service = new ProductService();
ArrayList<ProductVo> list = service.getAll(); // 전체검색
request.setAttribute("list", list); // request에 결과 담기
// 뷰페이지에 보여주기
RequestDispatcher dis = request.getRequestDispatcher("/product/list.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>webApp2</h3>
<a href="/webApp2/Join">회원가입</a><br/>
<!-- 5. 회원가입2 -->
<a href="/webApp2/test/Join">회원가입2</a><br/>
<!-- 4. 로그인 -- /test/Login에 get방식 요청 -->
<a href = "/webApp2/test/Login">로그인</a><br/>
<!-- 3. id 검색 -->
<form action="/webApp2/MyInfo" method="post">
검색할 id:<input type="text" name="id">
<input type="submit" value = "검색">
</form><br/>
<!-- 7. 상품 전체 목록 -->
<a href="/webApp2/product/list">상품목록</a><br/>
</body>
</html>
add.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 입력</h3>
<form action = "/webApp2/product/add" method = "post">
<table border = "1">
<tr><th>제품명</th><td><input type = "text" name = "name"></td></tr>
<tr><th>가격</th><td><input type = "number" name = "price"></td></tr>
<tr><th>수량</th><td><input type = "number" name = "amount"></td></tr>
<tr><th>추가</th><td><input type = "submit" value = "add"></td></tr>
</table>
</form>
</body>
</html>
list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%> <!-- page: jsp 설정 지시자 -->
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!-- 자바코드를 쓰면 너무 지저분하니까 라이브러리로 만들어놓음! -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>상품 목록</h3>
<!-- 6. 상품 추가 -->
<a href="/webApp2/product/add">상품추가</a><br/>
<!-- 홈화면 돌아가기 -->
<a href="/webApp2/index.jsp">홈화면</a><br/>
<table border="1">
<tr><th>제품번호</th><th>제품명</th><th>가격</th><th>수량</th></tr>
<!-- for문 - 자동으로 list.size 만큼 루프돎. 자동으로 "vo"에 넣어줌. -->
<!-- items = "리스트 or 배열" -->
<!-- ProductVo vo = list.get(i) 와 같음. -->
<c:forEach var="vo" items="${list }">
<tr><td>${vo.num}</td><td>${vo.name}</td><td>${vo.price}</td><td>${vo.amount}</td>
</c:forEach>
</table>
</form>
</body>
</html>