익명 게시판처럼 아무 이름으로 글 쓸 수 있음
1. 글작성
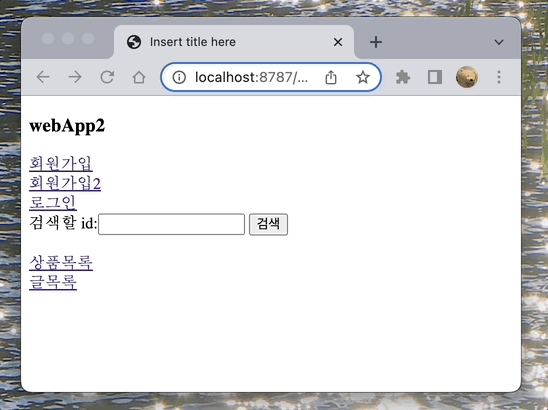
index.jsp
- 하이퍼 링크 타고 List.java로 이동
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>webApp2</h3>
<a href="/webApp2/Join">회원가입</a><br/>
<a href="/webApp2/test/Join">회원가입2</a><br/>
<a href = "/webApp2/test/Login">로그인</a><br/>
<form action="/webApp2/MyInfo" method="post">
검색할 id:<input type="text" name="id">
<input type="submit" value = "검색">
</form><br/>
<a href="/webApp2/product/list">상품목록</a><br/>
<!-- 방명록 -->
<a href="/webApp2/guest/list">글목록</a><br/>
</body>
</html>
List.java
- doGet() -- Service 메소드로 전체검색 -> 결과값을 request에 담음 -> 뷰페이지(list.jsp)로 이동
package guestbook.controller;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class List
*/
@WebServlet(name = "List2", urlPatterns = { "/guest/list" })
public class List extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public List() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html; charset=UTF-8");
// service로 기능 실행
GuestBookService service = new GuestBookService();
ArrayList<GuestBookVo> list = service.getAll(); // 전체검색
// 결과값을 request에 담음
request.setAttribute("list", list);
// 뷰페이지로 이동
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/list.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
list.jsp
- jstl의 c:forEach 문으로 리스트 값을 하나하나 출력해 table로 보여줌.
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><a href = "/webApp2/guest/detail?num=${vo.num }">${vo.content}</a></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
- 글번호 검색 (-> Get.java) / 작성자 검색 (-> GetByWriter.java) 을 할 수 있음.
- 글작성 버튼을 누르면 Add.java로 이동
<input type="button" value="글작성"
onclick="location.href = '/webApp2/guest/add'">
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a() {
let type = f.searchType.value;
let span = document.getElementById("searchSpan");
let html = "";
if (type == "1") {
html = "<input type = 'number' name = 'num'>";
f.action = "/webApp2/guest/get";
} else {
html = "<input type = 'text' name = 'writer'>";
f.action = "/webApp2/guest/getbywriter"; //form의 액션 속성에 값 넣어줌
}
span.innerHTML = html;
}
</script>
</head>
<body>
<h3>글목록</h3>
<input type="button" value="글작성"
onclick="location.href = '/webApp2/guest/add'">
<input type="button" value="홈화면"
onclick="location.href = '/webApp2/index.jsp'">
<span><form action="/webApp2/guest/get" method="post" name="f">
<span> <select name="searchType" onchange="a()">
<option value="1">글번호</option>
<option value="2">작성자</option>
</select>
</span> <span id="searchSpan"><input type='number' name='num'></span>
<input type="submit" value="검색">
</form></span>
<table border="1">
<tr>
<th>글번호</th>
<th>작성자</th>
<th>작성일</th>
<th>내용</th>
<th>조회수</th>
</tr>
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><a href = "/webApp2/guest/detail?num=${vo.num }">${vo.content}</a></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
Add.java
- doGet() -- 글작성 폼(add.jsp)으로 이동
- doPost() -- add.jsp에 입력한 값을 Service의 추가 메소드로 처리함 -> List.java로 이동해 리프레시한 글목록 보여줌
package guestbook.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class Add
*/
@WebServlet(name = "Add2", urlPatterns = { "/guest/add" })
public class Add extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Add() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/add.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
// 한글 안깨지게!
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String writer = request.getParameter("writer");
String pwd = request.getParameter("pwd");
String content = request.getParameter("content");
GuestBookService service = new GuestBookService();
service.addBook(new GuestBookVo(0, writer, null, pwd, content, 0));
response.sendRedirect("/webApp2/guest/list");
}
}
add.jsp
- 글 작성 table을 보여줌.
- 취소 버튼을 누르면 List.java로 이동. 뒤로가기 됨.
- 작성 완료 버튼을 누르면 submit 되고 Add.java의 doPost() 실행됨
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action = "/webApp2/guest/add" method = "post">
<table border = "1">
<tr><th>작성자</th><td><input type = "text" name = "writer"></td></tr>
<tr><th>글비밀번호</th><td><input type = "password" name = "pwd"></td></tr>
<tr><th>내용</th><td><input type = "text" name = "content"></td></tr>
<tr><td><input type="button" value="취소" onclick="location.href='/webApp2/guest/list'"></td>
<td><input type="submit" value="작성완료"></td></tr>
</table>
</form>
</body>
</html>
2-1. 글 내용 눌러서 상세페이지 들어가기 + 수정/삭제
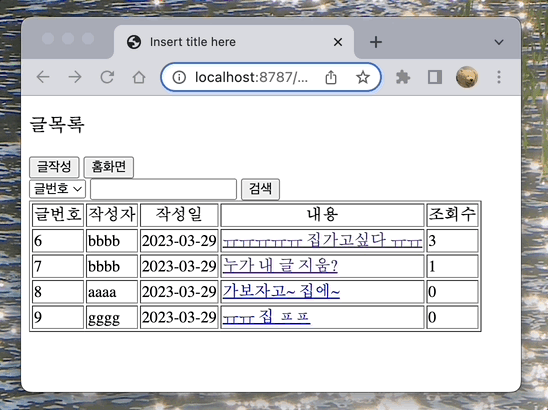
글 수정 및 삭제를 하는 방법은 두가지.
1) 글목록 - 글 내용 링크를 눌러서 -> 글 상세페이지 들어가서 -> 수정/삭제 버튼 누르기
2) 글 번호로 검색해서 -> 글 상세페이지 들어가서 -> 수정/삭제 버튼 누르기
1) 글 내용 링크를 눌러서 글 상세 페이지에 들어가려면,
글목록(list.jsp)에서 글 내용 하이퍼링크를 누르면 Detail.java로 이동.
Detail.java
- doGet() -- url과 함께 전송된 글 번호를 읽어와서 -> Service 메소드 호출 (조회수 올리기, 글번호로 검색)
-> 결과값 담아서 -> 상세페이지 폼(detail.jsp)으로 이동
- doPost() -- detail.jsp에서 수정버튼을 누르면 -> 글번호와 수정한 내용을 읽어와서
-> Service의 수정메소드 호출 -> List.java로 이동해 리프레시한 글목록 보여줌
package guestbook.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class Detail
*/
@WebServlet(name = "Detail2", urlPatterns = { "/guest/detail" })
public class Detail extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Detail() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
int num = Integer.parseInt(request.getParameter("num")); // url 주소로 보낸 num값 읽어오기
GuestBookService service = new GuestBookService();
service.editCnt(num); // 조회수 올리기
GuestBookVo vo = service.getBook(num); // 번호로 검색
request.setAttribute("vo", vo); // 검색한 결과값 담기 (jsp에서 쓸 수 있게)
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/detail.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html; charset=UTF-8");
int num = Integer.parseInt(request.getParameter("num"));
String content = request.getParameter("content");
GuestBookService service = new GuestBookService();
service.editBook(new GuestBookVo (num, "", null, "", content, 0));
response.sendRedirect("/webApp2/guest/list");
}
}
detail.jsp
- 비밀번호를 제외한 글 관련 정보를 table에 담아 보여줌
- 수정할 수 있는 내용 빼고는 readonly로 해 읽기만 하게 함
- 수정 버튼 -> prompt로 글 비밀번호 값을 받고 값이 일치하면 Detail.java 로 submit 해 doPost() 호출.
<head>
<script>
function a(){
let pwd = prompt('글 비밀번호');
if (${vo.pwd}==pwd){
f.submit();
} else {
alert('땡~!')
}
}
</script>
</head>
<body>
<form action = "/webApp2/guest/detail" name="f" method = "post">
<input type="button" value="수정" onclick="a()">
</form>
</body>
- 삭제 버튼 -> prompt로 글 비밀번호 값을 받고 값이 일치하면 글 번호와 함께 Del.java로 이동.
<head>
<script>
function b() {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/del?num=${vo.num}";
if (${vo.pwd}==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<input type="button" value="삭제" onclick="b()">
</body>
</html>
- 뒤로가기 버튼 -> List.java로 이동해 글목록 보여줌
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a(){
let pwd = prompt('글 비밀번호');
if (${vo.pwd}==pwd){
f.submit();
} else {
alert('땡~!')
}
}
function b() {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/del?num=${vo.num}";
if (${vo.pwd}==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<form action = "/webApp2/guest/detail" name="f" method = "post">
<table border = "1">
<tr><th>글번호</th><td><input type = "number" value="${vo.num}" name = "num" readonly></td></tr>
<tr><th>작성자</th><td><input type = "text" value="${vo.writer}" readonly></td></tr>
<tr><th>작성일</th><td><input type = "text" value="${vo.wDate}" readonly></td></tr>
<tr><th>내용</th><td><input type = "text" value="${vo.content}" name = "content"></td></tr>
<tr><th>조회수</th><td><input type = "number" value="${vo.cnt}" readonly></td></tr>
</table>
<input type="button" value="수정" onclick="a()">
<input type="button" value="삭제" onclick="b()">
<input type="button" value="뒤로가기" onclick = "location.href='/webApp2/guest/list'">
</form>
</body>
</html>
Del.java
- doGet() -- url과 함께 전송된 글 번호를 읽어와서 -> Service 삭제 메소드 호출 -> List.java로 이동해 리프레시한 글목록 보여줌
package guestbook.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
/**
* Servlet implementation class Del
*/
@WebServlet("/guest/del")
public class Del extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Del() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
int num = Integer.parseInt(request.getParameter("num"));
GuestBookService service = new GuestBookService();
service.delBook(num);
response.sendRedirect("/webApp2/guest/list"); }
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
2-2. 글 번호로 검색해서 상세페이지 들어가기
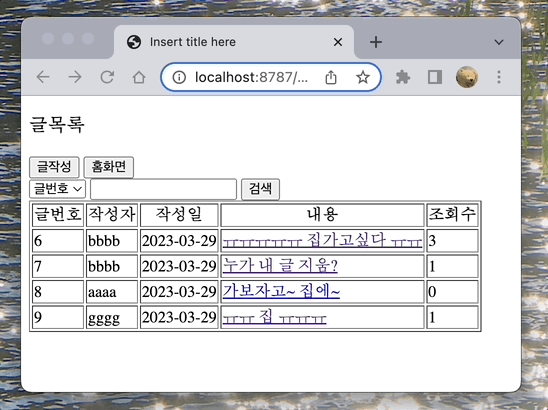
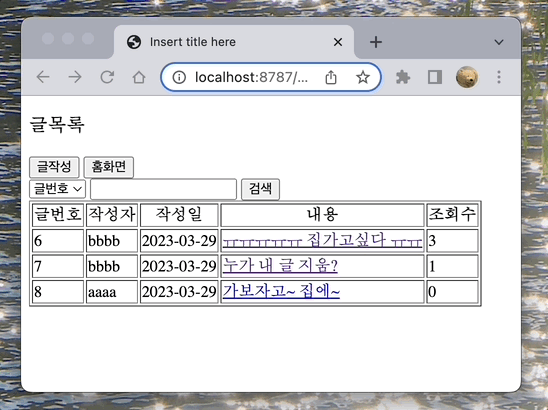
2) 글 번호로 검색해 글 상세 페이지에 들어가려면,
글목록(list.jsp)의 콤보박스를 글번호로 하고 검색창에 글 번호를 입력하면 Get.java로 이동
<script>
function a() {
let type = f.searchType.value;
let span = document.getElementById("searchSpan");
let html = "";
if (type == "1") {
html = "<input type = 'number' name = 'num'>";
f.action = "/webApp2/guest/get";
} else {
html = "<input type = 'text' name = 'writer'>";
f.action = "/webApp2/guest/getbywriter"; //form의 액션 속성에 값 넣어줌
}
span.innerHTML = html;
}
</script>
</head>
<body>
<span><form action="/webApp2/guest/get" method="post" name="f">
<span> <select name="searchType" onchange="a()">
<option value="1">글번호</option>
<option value="2">작성자</option>
</select>
</span> <span id="searchSpan"><input type='number' name='num'></span>
<input type="submit" value="검색">
</form></span>
</body>
Get.java
- doGet() --검색한 글 번호를 읽어와서 -> Service 검색 메소드 호출 -> 객체 담아 detail.jsp로 이동해 상세페이지 보여줌.
-- detail.jsp는 글 하나만 보여주도록 되어 있음.
package guestbook.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class Get
*/
@WebServlet("/guest/get")
public class Get extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Get() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
int num = Integer.parseInt(request.getParameter("num"));
GuestBookService service = new GuestBookService();
GuestBookVo vo = service.getBook(num);
request.setAttribute("vo", vo);
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/detail.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
글목록(list.jsp)의 콤보박스를 작성자로 하고 검색창에 작성자를 입력하면 GetByWriter.java로 이동
GetByWriter.java
- doGet() -- 검색한 작성자를 읽어와서 -> Service 작성자검색 메소드 호출 -> 리스트에 담아 글목록(list.jsp)으로 이동
-- list.jsp는 리스트에 들어있는 객체를 하나씩 꺼내서 보여주도록 되어있음.
package guestbook.controller;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class GetByWriter
*/
@WebServlet("/guest/getbywriter")
public class GetByWriter extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public GetByWriter() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String writer = request.getParameter("writer");
GuestBookService service = new GuestBookService();
ArrayList<GuestBookVo> list = service.getByWriter(writer);
request.setAttribute("list", list);
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/list.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
VO
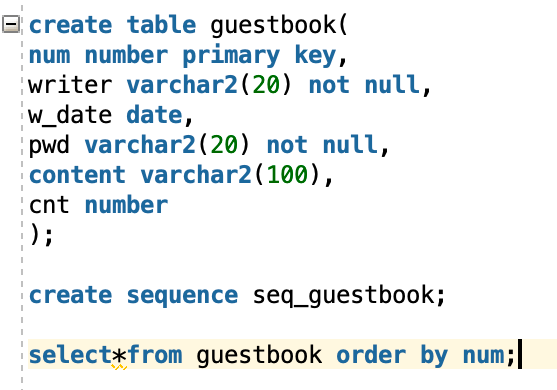
글번호 / seq_guestbook.nextval
작성자 /
작성일 /
글비밀번호 /
내용 /
조회수 / 상세페이지 들어갈 때마다 숫자 ++
package guestbook;
import java.sql.Date;
public class GuestBookVo {
private int num;
private String writer;
private Date wDate;
private String pwd;
private String content;
private int cnt; // 조회수
public GuestBookVo() {}
public GuestBookVo(int num, String writer, Date wDate, String pwd, String content, int cnt) {
super();
this.num = num;
this.writer = writer;
this.wDate = wDate;
this.pwd = pwd;
this.content = content;
this.cnt = cnt;
}
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
public String getWriter() {
return writer;
}
public void setWriter(String writer) {
this.writer = writer;
}
public Date getwDate() {
return wDate;
}
public void setwDate(Date wDate) {
this.wDate = wDate;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public int getCnt() {
return cnt;
}
public void setCnt(int cnt) {
this.cnt = cnt;
}
@Override
public String toString() {
return "GuestbookVo [num=" + num + ", writer=" + writer + ", wDate=" + wDate + ", pwd=" + pwd + ", content="
+ content + ", cnt=" + cnt + "]";
}
}
DAO
글작성, public void insert(GuestBookVo vo){}
번호로 검색, public GuestBookVo select(int num){}
작성자로 검색, public ArrayList<GuestBookVo> selectByWriter(String writer){}
전체목록, public ArrayList<G selectAll(){}
글 내용 수정, public void update(GuestBookVo vo){}
조회수 올리기, public void updateCnt(int num){}
// "update guestbook set cnt=cnt+1 where num = ?"
글삭제, public void delete(int num){}
package guestbook;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import conn.DBConnect;
public class GuestBookDao {
private DBConnect dbconn;
public GuestBookDao() {
dbconn = DBConnect.getInstance();
}
// 글작성,
public void insert(GuestBookVo vo) {
Connection conn = dbconn.conn();
String sql = "insert into guestbook values(seq_guestbook.nextval, ?, sysdate, ?, ?, 0)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, vo.getWriter());
pstmt.setString(2, vo.getPwd());
pstmt.setString(3, vo.getContent());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 추가되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 번호로 검색,
public GuestBookVo select(int num) {
Connection conn = dbconn.conn();
String sql = "select * from guestbook where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
if (rs.next()) { // 검색결과가 있으면 실행
return new GuestBookVo(rs.getInt(1), rs.getString(2), rs.getDate(3), rs.getString(4), rs.getString(5), rs.getInt(6));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return null; // 검색된거 없으면 널값 리턴
}
// 작성자로 검색,
public ArrayList<GuestBookVo> selectByWriter(String writer) {
ArrayList<GuestBookVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from guestbook where writer = ? order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, writer);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
while (rs.next()) { // 검색결과가 있으면 실행
list.add(new GuestBookVo(rs.getInt(1), rs.getString(2), rs.getDate(3), rs.getString(4), rs.getString(5), rs.getInt(6)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 전체목록,
public ArrayList<GuestBookVo> selectAll() {
ArrayList<GuestBookVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from guestbook order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
while (rs.next()) { // 검색결과가 있으면 실행
list.add(new GuestBookVo(rs.getInt(1), rs.getString(2), rs.getDate(3), rs.getString(4), rs.getString(5), rs.getInt(6)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 글 내용 수정,
public void update(GuestBookVo vo) {
Connection conn = dbconn.conn();
String sql = "update guestbook set content = ? where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, vo.getContent());
pstmt.setInt(2, vo.getNum());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 수정되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 조회수 올리기,
public void updateCnt(int num){
Connection conn = dbconn.conn();
String sql = "update guestbook set cnt = cnt+1 where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
int number = pstmt.executeUpdate();
System.out.println("조회수" + number + "증가");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 글삭제,
public void delete(int num) {
Connection conn = dbconn.conn();
String sql = "delete guestbook where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
int number = pstmt.executeUpdate();
System.out.println(number + "줄이 삭제되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
Service
public void addBook(GuestBookVo vo){}
public GuestBookVo getBook(int num){}
public ArrayList<GuestBookVo> getByWriter(String writer) {}
public ArrayList<GuestBookVo> getAll(){}
public void editBook(GuestBookVo vo){} // 글 내용 수정
publiv void delBook(int num){}
package guestbook;
import java.util.ArrayList;
public class GuestBookService {
private GuestBookDao dao;
public GuestBookService() {
dao = new GuestBookDao();
}
public void addBook(GuestBookVo vo){
dao.insert(vo);
}
public GuestBookVo getBook(int num){
return dao.select(num);
}
public ArrayList<GuestBookVo> getByWriter(String writer) {
return dao.selectByWriter(writer);
}
public ArrayList<GuestBookVo> getAll(){
return dao.selectAll();
}
// 글 내용 수정
public void editBook(GuestBookVo vo){
dao.update(vo);
}
public void editCnt(int num) {
dao.updateCnt(num);
}
public void delBook(int num){
dao.delete(num);
}
}
<url>
/guest/list : 글목록
/guest/add (get) : 글 작성폼
/guest/add (post) : 글 작성완료 => 글목록으로 이동
/guest/get : 글 번호로 검색
=> 수정버튼 클릭 => prompt로 글 비밀번호 물어봄
=> 일치 => 수정폼으로 이동
=> 불일치 => alert('틀렸습니다')
/guest/getbywriter : 작성자로 검색
/guest/edit (get) : 수정폼
/guest/edit (post) : 수정완료 => 글목록으로 이동
/guest/del : 삭제
(아쉬운 코드) 글 내용 누르면 비밀번호창 뜨고, 비밀번호 맞으면 들어가서 수정, 삭제 할 수 있음
-- 글 작성자만 볼 수 있기 때문에 조회수가 의미 없음
-- 루프 문에서의 해당 글 번호를 호출해야 하기 때문에, 함수 호출할 때 파라메터로 값을 받아야 함.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<script>
function b(num, pwd2) {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/detail?num="+num;
if (pwd2==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<table border="1">
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><span onclick='b(${vo.num}, "${vo.pwd }")'>${vo.content}</span></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a() {
let type = f.searchType.value;
let span = document.getElementById("searchSpan");
let html = "";
if (type == "1") {
html = "<input type = 'number' name = 'num'>";
f.action = "/webApp2/product/get";
} else {
html = "<input type = 'text' name = 'writer'>";
f.action = "/webApp2/product/getbywriter"; //form의 액션 속성에 값 넣어줌
}
span.innerHTML = html;
}
function b(num, pwd2) {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/detail?num="+num;
if (pwd2==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<h3>글목록</h3>
<form action="/webApp2/product/getbyname" method="post" name="f">
<span> <select name="searchType" onchange="a()">
<option value="1">글번호</option>
<option value="2">작성자</option>
</select>
</span> <span id="searchSpan"><input type='number' name='num'></span>
<input type="submit" value="검색">
</form>
<input type="button" value="글작성"
onclick="location.href = '/webApp2/guest/add'">
<br />
<table border="1">
<tr>
<th>글번호</th>
<th>작성자</th>
<th>작성일</th>
<th>내용</th>
<th>조회수</th>
</tr>
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><span onclick='b(${vo.num}, "${vo.pwd }")'>${vo.content}</span></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
익명 게시판처럼 아무 이름으로 글 쓸 수 있음
1. 글작성
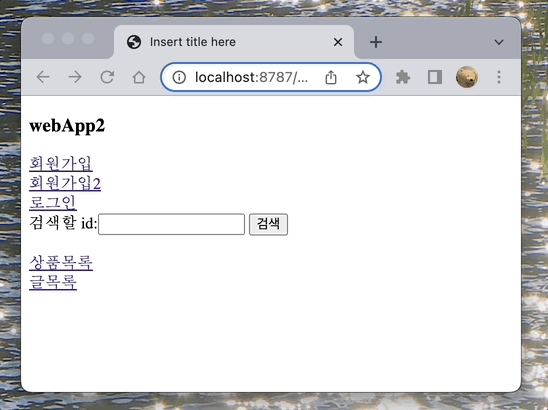
index.jsp
- 하이퍼 링크 타고 List.java로 이동
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>webApp2</h3>
<a href="/webApp2/Join">회원가입</a><br/>
<a href="/webApp2/test/Join">회원가입2</a><br/>
<a href = "/webApp2/test/Login">로그인</a><br/>
<form action="/webApp2/MyInfo" method="post">
검색할 id:<input type="text" name="id">
<input type="submit" value = "검색">
</form><br/>
<a href="/webApp2/product/list">상품목록</a><br/>
<!-- 방명록 -->
<a href="/webApp2/guest/list">글목록</a><br/>
</body>
</html>
List.java
- doGet() -- Service 메소드로 전체검색 -> 결과값을 request에 담음 -> 뷰페이지(list.jsp)로 이동
package guestbook.controller;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class List
*/
@WebServlet(name = "List2", urlPatterns = { "/guest/list" })
public class List extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public List() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html; charset=UTF-8");
// service로 기능 실행
GuestBookService service = new GuestBookService();
ArrayList<GuestBookVo> list = service.getAll(); // 전체검색
// 결과값을 request에 담음
request.setAttribute("list", list);
// 뷰페이지로 이동
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/list.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
list.jsp
- jstl의 c:forEach 문으로 리스트 값을 하나하나 출력해 table로 보여줌.
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><a href = "/webApp2/guest/detail?num=${vo.num }">${vo.content}</a></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
- 글번호 검색 (-> Get.java) / 작성자 검색 (-> GetByWriter.java) 을 할 수 있음.
- 글작성 버튼을 누르면 Add.java로 이동
<input type="button" value="글작성"
onclick="location.href = '/webApp2/guest/add'">
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a() {
let type = f.searchType.value;
let span = document.getElementById("searchSpan");
let html = "";
if (type == "1") {
html = "<input type = 'number' name = 'num'>";
f.action = "/webApp2/guest/get";
} else {
html = "<input type = 'text' name = 'writer'>";
f.action = "/webApp2/guest/getbywriter"; //form의 액션 속성에 값 넣어줌
}
span.innerHTML = html;
}
</script>
</head>
<body>
<h3>글목록</h3>
<input type="button" value="글작성"
onclick="location.href = '/webApp2/guest/add'">
<input type="button" value="홈화면"
onclick="location.href = '/webApp2/index.jsp'">
<span><form action="/webApp2/guest/get" method="post" name="f">
<span> <select name="searchType" onchange="a()">
<option value="1">글번호</option>
<option value="2">작성자</option>
</select>
</span> <span id="searchSpan"><input type='number' name='num'></span>
<input type="submit" value="검색">
</form></span>
<table border="1">
<tr>
<th>글번호</th>
<th>작성자</th>
<th>작성일</th>
<th>내용</th>
<th>조회수</th>
</tr>
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><a href = "/webApp2/guest/detail?num=${vo.num }">${vo.content}</a></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
Add.java
- doGet() -- 글작성 폼(add.jsp)으로 이동
- doPost() -- add.jsp에 입력한 값을 Service의 추가 메소드로 처리함 -> List.java로 이동해 리프레시한 글목록 보여줌
package guestbook.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class Add
*/
@WebServlet(name = "Add2", urlPatterns = { "/guest/add" })
public class Add extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Add() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/add.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
// 한글 안깨지게!
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String writer = request.getParameter("writer");
String pwd = request.getParameter("pwd");
String content = request.getParameter("content");
GuestBookService service = new GuestBookService();
service.addBook(new GuestBookVo(0, writer, null, pwd, content, 0));
response.sendRedirect("/webApp2/guest/list");
}
}
add.jsp
- 글 작성 table을 보여줌.
- 취소 버튼을 누르면 List.java로 이동. 뒤로가기 됨.
- 작성 완료 버튼을 누르면 submit 되고 Add.java의 doPost() 실행됨
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action = "/webApp2/guest/add" method = "post">
<table border = "1">
<tr><th>작성자</th><td><input type = "text" name = "writer"></td></tr>
<tr><th>글비밀번호</th><td><input type = "password" name = "pwd"></td></tr>
<tr><th>내용</th><td><input type = "text" name = "content"></td></tr>
<tr><td><input type="button" value="취소" onclick="location.href='/webApp2/guest/list'"></td>
<td><input type="submit" value="작성완료"></td></tr>
</table>
</form>
</body>
</html>
2-1. 글 내용 눌러서 상세페이지 들어가기 + 수정/삭제
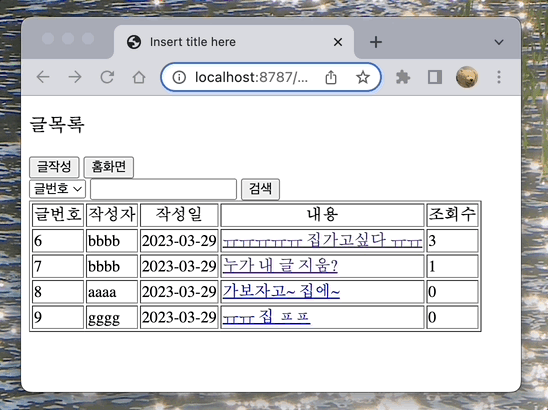
글 수정 및 삭제를 하는 방법은 두가지.
1) 글목록 - 글 내용 링크를 눌러서 -> 글 상세페이지 들어가서 -> 수정/삭제 버튼 누르기
2) 글 번호로 검색해서 -> 글 상세페이지 들어가서 -> 수정/삭제 버튼 누르기
1) 글 내용 링크를 눌러서 글 상세 페이지에 들어가려면,
글목록(list.jsp)에서 글 내용 하이퍼링크를 누르면 Detail.java로 이동.
Detail.java
- doGet() -- url과 함께 전송된 글 번호를 읽어와서 -> Service 메소드 호출 (조회수 올리기, 글번호로 검색)
-> 결과값 담아서 -> 상세페이지 폼(detail.jsp)으로 이동
- doPost() -- detail.jsp에서 수정버튼을 누르면 -> 글번호와 수정한 내용을 읽어와서
-> Service의 수정메소드 호출 -> List.java로 이동해 리프레시한 글목록 보여줌
package guestbook.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class Detail
*/
@WebServlet(name = "Detail2", urlPatterns = { "/guest/detail" })
public class Detail extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Detail() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
int num = Integer.parseInt(request.getParameter("num")); // url 주소로 보낸 num값 읽어오기
GuestBookService service = new GuestBookService();
service.editCnt(num); // 조회수 올리기
GuestBookVo vo = service.getBook(num); // 번호로 검색
request.setAttribute("vo", vo); // 검색한 결과값 담기 (jsp에서 쓸 수 있게)
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/detail.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html; charset=UTF-8");
int num = Integer.parseInt(request.getParameter("num"));
String content = request.getParameter("content");
GuestBookService service = new GuestBookService();
service.editBook(new GuestBookVo (num, "", null, "", content, 0));
response.sendRedirect("/webApp2/guest/list");
}
}
detail.jsp
- 비밀번호를 제외한 글 관련 정보를 table에 담아 보여줌
- 수정할 수 있는 내용 빼고는 readonly로 해 읽기만 하게 함
- 수정 버튼 -> prompt로 글 비밀번호 값을 받고 값이 일치하면 Detail.java 로 submit 해 doPost() 호출.
<head>
<script>
function a(){
let pwd = prompt('글 비밀번호');
if (${vo.pwd}==pwd){
f.submit();
} else {
alert('땡~!')
}
}
</script>
</head>
<body>
<form action = "/webApp2/guest/detail" name="f" method = "post">
<input type="button" value="수정" onclick="a()">
</form>
</body>
- 삭제 버튼 -> prompt로 글 비밀번호 값을 받고 값이 일치하면 글 번호와 함께 Del.java로 이동.
<head>
<script>
function b() {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/del?num=${vo.num}";
if (${vo.pwd}==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<input type="button" value="삭제" onclick="b()">
</body>
</html>
- 뒤로가기 버튼 -> List.java로 이동해 글목록 보여줌
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a(){
let pwd = prompt('글 비밀번호');
if (${vo.pwd}==pwd){
f.submit();
} else {
alert('땡~!')
}
}
function b() {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/del?num=${vo.num}";
if (${vo.pwd}==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<form action = "/webApp2/guest/detail" name="f" method = "post">
<table border = "1">
<tr><th>글번호</th><td><input type = "number" value="${vo.num}" name = "num" readonly></td></tr>
<tr><th>작성자</th><td><input type = "text" value="${vo.writer}" readonly></td></tr>
<tr><th>작성일</th><td><input type = "text" value="${vo.wDate}" readonly></td></tr>
<tr><th>내용</th><td><input type = "text" value="${vo.content}" name = "content"></td></tr>
<tr><th>조회수</th><td><input type = "number" value="${vo.cnt}" readonly></td></tr>
</table>
<input type="button" value="수정" onclick="a()">
<input type="button" value="삭제" onclick="b()">
<input type="button" value="뒤로가기" onclick = "location.href='/webApp2/guest/list'">
</form>
</body>
</html>
Del.java
- doGet() -- url과 함께 전송된 글 번호를 읽어와서 -> Service 삭제 메소드 호출 -> List.java로 이동해 리프레시한 글목록 보여줌
package guestbook.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
/**
* Servlet implementation class Del
*/
@WebServlet("/guest/del")
public class Del extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Del() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
int num = Integer.parseInt(request.getParameter("num"));
GuestBookService service = new GuestBookService();
service.delBook(num);
response.sendRedirect("/webApp2/guest/list"); }
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
2-2. 글 번호로 검색해서 상세페이지 들어가기
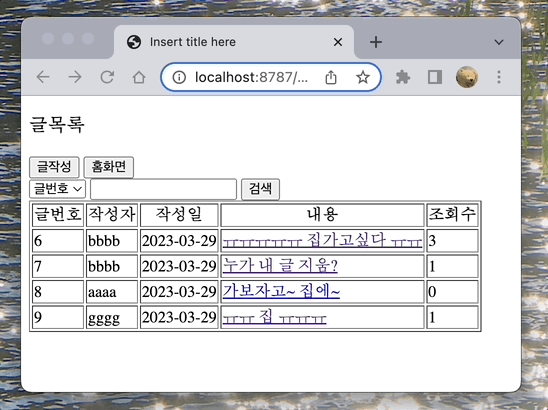
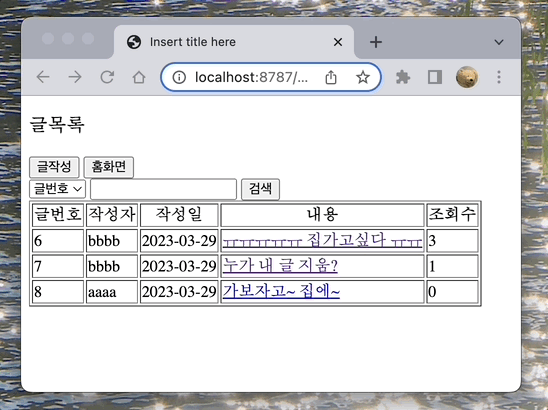
2) 글 번호로 검색해 글 상세 페이지에 들어가려면,
글목록(list.jsp)의 콤보박스를 글번호로 하고 검색창에 글 번호를 입력하면 Get.java로 이동
<script>
function a() {
let type = f.searchType.value;
let span = document.getElementById("searchSpan");
let html = "";
if (type == "1") {
html = "<input type = 'number' name = 'num'>";
f.action = "/webApp2/guest/get";
} else {
html = "<input type = 'text' name = 'writer'>";
f.action = "/webApp2/guest/getbywriter"; //form의 액션 속성에 값 넣어줌
}
span.innerHTML = html;
}
</script>
</head>
<body>
<span><form action="/webApp2/guest/get" method="post" name="f">
<span> <select name="searchType" onchange="a()">
<option value="1">글번호</option>
<option value="2">작성자</option>
</select>
</span> <span id="searchSpan"><input type='number' name='num'></span>
<input type="submit" value="검색">
</form></span>
</body>
Get.java
- doGet() --검색한 글 번호를 읽어와서 -> Service 검색 메소드 호출 -> 객체 담아 detail.jsp로 이동해 상세페이지 보여줌.
-- detail.jsp는 글 하나만 보여주도록 되어 있음.
package guestbook.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class Get
*/
@WebServlet("/guest/get")
public class Get extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Get() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
int num = Integer.parseInt(request.getParameter("num"));
GuestBookService service = new GuestBookService();
GuestBookVo vo = service.getBook(num);
request.setAttribute("vo", vo);
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/detail.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
글목록(list.jsp)의 콤보박스를 작성자로 하고 검색창에 작성자를 입력하면 GetByWriter.java로 이동
GetByWriter.java
- doGet() -- 검색한 작성자를 읽어와서 -> Service 작성자검색 메소드 호출 -> 리스트에 담아 글목록(list.jsp)으로 이동
-- list.jsp는 리스트에 들어있는 객체를 하나씩 꺼내서 보여주도록 되어있음.
package guestbook.controller;
import java.io.IOException;
import java.util.ArrayList;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import guestbook.GuestBookService;
import guestbook.GuestBookVo;
/**
* Servlet implementation class GetByWriter
*/
@WebServlet("/guest/getbywriter")
public class GetByWriter extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public GetByWriter() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String writer = request.getParameter("writer");
GuestBookService service = new GuestBookService();
ArrayList<GuestBookVo> list = service.getByWriter(writer);
request.setAttribute("list", list);
RequestDispatcher dis = request.getRequestDispatcher("/guestbook/list.jsp");
dis.forward(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
VO
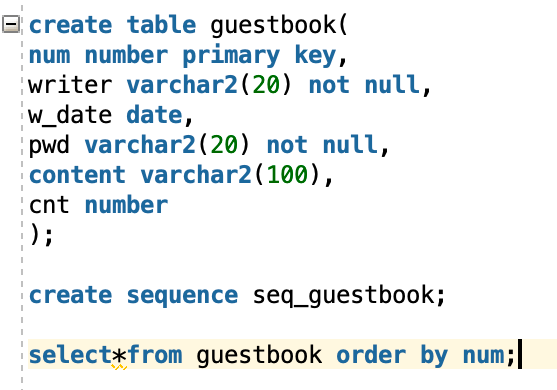
글번호 / seq_guestbook.nextval
작성자 /
작성일 /
글비밀번호 /
내용 /
조회수 / 상세페이지 들어갈 때마다 숫자 ++
package guestbook;
import java.sql.Date;
public class GuestBookVo {
private int num;
private String writer;
private Date wDate;
private String pwd;
private String content;
private int cnt; // 조회수
public GuestBookVo() {}
public GuestBookVo(int num, String writer, Date wDate, String pwd, String content, int cnt) {
super();
this.num = num;
this.writer = writer;
this.wDate = wDate;
this.pwd = pwd;
this.content = content;
this.cnt = cnt;
}
public int getNum() {
return num;
}
public void setNum(int num) {
this.num = num;
}
public String getWriter() {
return writer;
}
public void setWriter(String writer) {
this.writer = writer;
}
public Date getwDate() {
return wDate;
}
public void setwDate(Date wDate) {
this.wDate = wDate;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public int getCnt() {
return cnt;
}
public void setCnt(int cnt) {
this.cnt = cnt;
}
@Override
public String toString() {
return "GuestbookVo [num=" + num + ", writer=" + writer + ", wDate=" + wDate + ", pwd=" + pwd + ", content="
+ content + ", cnt=" + cnt + "]";
}
}
DAO
글작성, public void insert(GuestBookVo vo){}
번호로 검색, public GuestBookVo select(int num){}
작성자로 검색, public ArrayList<GuestBookVo> selectByWriter(String writer){}
전체목록, public ArrayList<G selectAll(){}
글 내용 수정, public void update(GuestBookVo vo){}
조회수 올리기, public void updateCnt(int num){}
// "update guestbook set cnt=cnt+1 where num = ?"
글삭제, public void delete(int num){}
package guestbook;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import conn.DBConnect;
public class GuestBookDao {
private DBConnect dbconn;
public GuestBookDao() {
dbconn = DBConnect.getInstance();
}
// 글작성,
public void insert(GuestBookVo vo) {
Connection conn = dbconn.conn();
String sql = "insert into guestbook values(seq_guestbook.nextval, ?, sysdate, ?, ?, 0)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, vo.getWriter());
pstmt.setString(2, vo.getPwd());
pstmt.setString(3, vo.getContent());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 추가되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 번호로 검색,
public GuestBookVo select(int num) {
Connection conn = dbconn.conn();
String sql = "select * from guestbook where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
if (rs.next()) { // 검색결과가 있으면 실행
return new GuestBookVo(rs.getInt(1), rs.getString(2), rs.getDate(3), rs.getString(4), rs.getString(5), rs.getInt(6));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return null; // 검색된거 없으면 널값 리턴
}
// 작성자로 검색,
public ArrayList<GuestBookVo> selectByWriter(String writer) {
ArrayList<GuestBookVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from guestbook where writer = ? order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, writer);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
while (rs.next()) { // 검색결과가 있으면 실행
list.add(new GuestBookVo(rs.getInt(1), rs.getString(2), rs.getDate(3), rs.getString(4), rs.getString(5), rs.getInt(6)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 전체목록,
public ArrayList<GuestBookVo> selectAll() {
ArrayList<GuestBookVo> list = new ArrayList<>();
Connection conn = dbconn.conn();
String sql = "select * from guestbook order by num";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery(); // 검색결과 넣기
while (rs.next()) { // 검색결과가 있으면 실행
list.add(new GuestBookVo(rs.getInt(1), rs.getString(2), rs.getDate(3), rs.getString(4), rs.getString(5), rs.getInt(6)));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return list;
}
// 글 내용 수정,
public void update(GuestBookVo vo) {
Connection conn = dbconn.conn();
String sql = "update guestbook set content = ? where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, vo.getContent());
pstmt.setInt(2, vo.getNum());
int num = pstmt.executeUpdate();
System.out.println(num + "줄이 수정되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 조회수 올리기,
public void updateCnt(int num){
Connection conn = dbconn.conn();
String sql = "update guestbook set cnt = cnt+1 where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
int number = pstmt.executeUpdate();
System.out.println("조회수" + number + "증가");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
// 글삭제,
public void delete(int num) {
Connection conn = dbconn.conn();
String sql = "delete guestbook where num = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, num);
int number = pstmt.executeUpdate();
System.out.println(number + "줄이 삭제되었습니다.");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
Service
public void addBook(GuestBookVo vo){}
public GuestBookVo getBook(int num){}
public ArrayList<GuestBookVo> getByWriter(String writer) {}
public ArrayList<GuestBookVo> getAll(){}
public void editBook(GuestBookVo vo){} // 글 내용 수정
publiv void delBook(int num){}
package guestbook;
import java.util.ArrayList;
public class GuestBookService {
private GuestBookDao dao;
public GuestBookService() {
dao = new GuestBookDao();
}
public void addBook(GuestBookVo vo){
dao.insert(vo);
}
public GuestBookVo getBook(int num){
return dao.select(num);
}
public ArrayList<GuestBookVo> getByWriter(String writer) {
return dao.selectByWriter(writer);
}
public ArrayList<GuestBookVo> getAll(){
return dao.selectAll();
}
// 글 내용 수정
public void editBook(GuestBookVo vo){
dao.update(vo);
}
public void editCnt(int num) {
dao.updateCnt(num);
}
public void delBook(int num){
dao.delete(num);
}
}
<url>
/guest/list : 글목록
/guest/add (get) : 글 작성폼
/guest/add (post) : 글 작성완료 => 글목록으로 이동
/guest/get : 글 번호로 검색
=> 수정버튼 클릭 => prompt로 글 비밀번호 물어봄
=> 일치 => 수정폼으로 이동
=> 불일치 => alert('틀렸습니다')
/guest/getbywriter : 작성자로 검색
/guest/edit (get) : 수정폼
/guest/edit (post) : 수정완료 => 글목록으로 이동
/guest/del : 삭제
(아쉬운 코드) 글 내용 누르면 비밀번호창 뜨고, 비밀번호 맞으면 들어가서 수정, 삭제 할 수 있음
-- 글 작성자만 볼 수 있기 때문에 조회수가 의미 없음
-- 루프 문에서의 해당 글 번호를 호출해야 하기 때문에, 함수 호출할 때 파라메터로 값을 받아야 함.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<script>
function b(num, pwd2) {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/detail?num="+num;
if (pwd2==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<table border="1">
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><span onclick='b(${vo.num}, "${vo.pwd }")'>${vo.content}</span></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a() {
let type = f.searchType.value;
let span = document.getElementById("searchSpan");
let html = "";
if (type == "1") {
html = "<input type = 'number' name = 'num'>";
f.action = "/webApp2/product/get";
} else {
html = "<input type = 'text' name = 'writer'>";
f.action = "/webApp2/product/getbywriter"; //form의 액션 속성에 값 넣어줌
}
span.innerHTML = html;
}
function b(num, pwd2) {
let pwd = prompt("글 비밀번호");
let url = "/webApp2/guest/detail?num="+num;
if (pwd2==pwd){
location.href=url;
} else {
alert("땡~!")
}
}
</script>
</head>
<body>
<h3>글목록</h3>
<form action="/webApp2/product/getbyname" method="post" name="f">
<span> <select name="searchType" onchange="a()">
<option value="1">글번호</option>
<option value="2">작성자</option>
</select>
</span> <span id="searchSpan"><input type='number' name='num'></span>
<input type="submit" value="검색">
</form>
<input type="button" value="글작성"
onclick="location.href = '/webApp2/guest/add'">
<br />
<table border="1">
<tr>
<th>글번호</th>
<th>작성자</th>
<th>작성일</th>
<th>내용</th>
<th>조회수</th>
</tr>
<c:forEach var="vo" items="${list }">
<tr>
<td>${vo.num}</td>
<td>${vo.writer}</td>
<td>${vo.wDate}</td>
<td><span onclick='b(${vo.num}, "${vo.pwd }")'>${vo.content}</span></td>
<td>${vo.cnt}</td>
</tr>
</c:forEach>
</table>
</body>
</html>