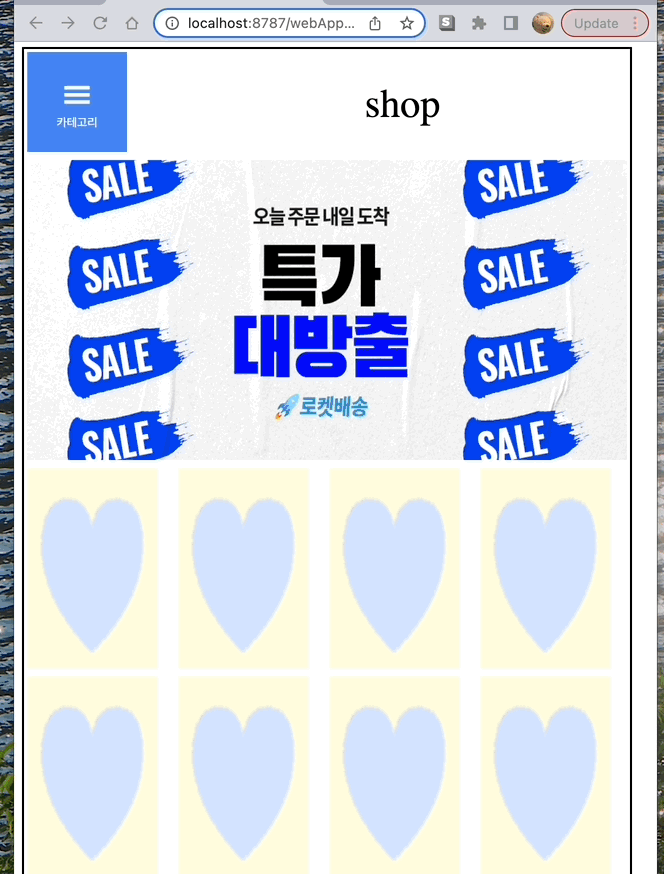
홈화면
- 대문 이미지 1초마다 변하게 하기
- 카테고리 이미지 누르면 (onmouseover, onmouseout) 대분류 카테고리 나오기
- 대분류 카테고리에서 항목에 마우스를 올리면 중분류 뜨게 하기
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style>
#cate1 {
position: absolute;
top: 112px;
left: 12px;
border: 1px solid blue;
background-color: white;
width: 100px;
height: 200px;
}
#cate2 {
position: absolute;
top: 112px;
left: 112px;
border: 1px solid blue;
background-color: white;
width: 100px;
height: 200px;
}
</style>
<script>
let sel1 = -1;
let cate2arr = [ [ "남성패션", "여성패션", "아동패션" ], [ "세탁기", "냉장고", "TV", "컴퓨터" ],
[ "침대", "옷장", "책상", "소파" ], [ "신발", "악세서리" ] ]; //중분류
let arr = [ "2.jpeg", "3.jpeg", "4.jpeg", "5.png", "1.jpg" ]; // 이미지 파일명
let cnt = 0;
function changeImg() {
let img = document.getElementById("img")
img.src = "../img/" + arr[cnt % 5]; //arr[0], arr[1]
cnt++;
setTimeout(changeImg, 1000); // 같은 함수명을 넣어서 계속 반복되게 함.
}
window.onload = function() {
changeImg();
}
function a() { /* onmouseover */
let cate1 = document.getElementById("cate1");
cate1.style.display = '';
}
function b(id) { /* onmouseout */
let cate = document.getElementById(id);
cate.style.display = 'none';
}
function c(idx) { // 대분류 항목에 마우스 올리면 중분류 나오게 하기
sel1 = idx;
let arr = cate2arr[idx]; // idx:0 [남성패션, 여성패션...]
let html = "";
for (i = 0; i < arr.length; i++) {
html += arr[i] + "<br/>";
}
let cate2 = document.getElementById("cate2");
cate2.innerHTML = html;
cate2.style.display = '';
}
function d() { // 중분류 배열 인덱스 초기화 (-1)
c(sel1);
}
</script>
</head>
<body>
<table style="border: 2px solid black">
<tr>
<td><img src="../img/cup_ca.png"
style="width: 100px; height: 100px" onmouseover="a()"
onmouseout="b('cate1')"></td>
<td colspan="3" style="font-size: 30pt; text-align: center">shop</td>
</tr>
<tr>
<td colspan="4"><img src="../img/1.jpg" id="img"
style="width: 600px; height: 300px"></td>
</tr>
<tr>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
</tr>
<tr>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
</tr>
</table>
<div onmouseover="a()" onmouseout="b('cate1')" id="cate1"
style="display: none;">
<table style="width: 100px">
<tr onmouseover="c(0)" onmouseout="b('cate2')">
<td>의류</td>
</tr>
<tr onmouseover="c(1)" onmouseout="b('cate2')">
<td>가전</td>
</tr>
<tr onmouseover="c(2)" onmouseout="b('cate2')">
<td>가구</td>
</tr>
<tr onmouseover="c(3)" onmouseout="b('cate2')">
<td>잡화</td>
</tr>
</table>
</div>
<div onmouseover="d()" onmouseout="b('cate2')" id="cate2"
style="display: none;"></div>
</body>
</html>
제품상세화면
- 홈화면에서 하단의 상품 이미지를 누르면 제품상세 html 페이지로 넘어가기
- 이미지에 마우스를 올리면(onmouseover) 이미지 변하게 하기
- 주문 수량에 따라 (주문수량 * 가격) 값을 결제 금액에 띄우기 (onchange)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a(el){ /* 마우스 올리면 이미지 전환 */
let bigImg = document.getElementById("bigImg");
bigImg.src = el.src;
}
function b(){ /* 주문수량*가격 값을 결제금액에 띄우기 (onchange) */
let price = parseInt(document.getElementById("price").innerHTML);
let amount = parseInt(document.getElementById("amount").value);
document.getElementById("total").innerHTML = price*amount;
}
</script>
</head>
<body>
<h3>제품 상세 페이지</h3>
<table border="1" cellspacing='0'>
<tr>
<td>
<table>
<tr>
<td colspan="4"><img id="bigImg" src="../img/b.png"
style="width: 200px; height: 200px"></td>
</tr>
<tr>
<td><img src="../img/a.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
<td><img src="../img/b.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
<td><img src="../img/a.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
<td><img src="../img/b.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
</tr>
</table>
</td>
<td>
<table border="1" cellspacing='0'>
<tr><th>제품명</th><td>파란 하트</td></tr>
<tr><th>가격</th><td id="price">30000</td></tr>
<tr><th>주문수량</th><td><input type="number" id="amount" onchange="b()"></td></tr>
<tr><th>결제금액</th><td id="total"></td></tr>
<tr><th>결제</th><td><input type="button" value="바로결제">
<input type="button" value="장바구니"></td></tr>
</table>
</td>
</tr>
<tr><td colspan = "2"> 상품 상세 설명</td></tr>
<tr>
<td colspan = "2"> 봄이 생각나는 하트 <br/>
<img src="../img/b.png" style="width:400px; height:400px">
</td>
</table>
</body>
</html>
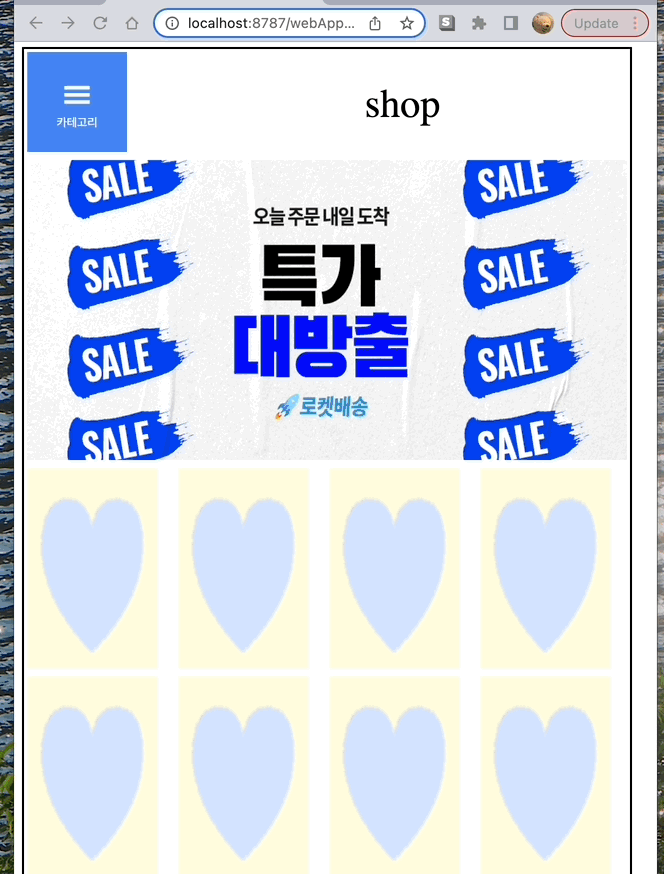
홈화면
- 대문 이미지 1초마다 변하게 하기
- 카테고리 이미지 누르면 (onmouseover, onmouseout) 대분류 카테고리 나오기
- 대분류 카테고리에서 항목에 마우스를 올리면 중분류 뜨게 하기
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style>
#cate1 {
position: absolute;
top: 112px;
left: 12px;
border: 1px solid blue;
background-color: white;
width: 100px;
height: 200px;
}
#cate2 {
position: absolute;
top: 112px;
left: 112px;
border: 1px solid blue;
background-color: white;
width: 100px;
height: 200px;
}
</style>
<script>
let sel1 = -1;
let cate2arr = [ [ "남성패션", "여성패션", "아동패션" ], [ "세탁기", "냉장고", "TV", "컴퓨터" ],
[ "침대", "옷장", "책상", "소파" ], [ "신발", "악세서리" ] ]; //중분류
let arr = [ "2.jpeg", "3.jpeg", "4.jpeg", "5.png", "1.jpg" ]; // 이미지 파일명
let cnt = 0;
function changeImg() {
let img = document.getElementById("img")
img.src = "../img/" + arr[cnt % 5]; //arr[0], arr[1]
cnt++;
setTimeout(changeImg, 1000); // 같은 함수명을 넣어서 계속 반복되게 함.
}
window.onload = function() {
changeImg();
}
function a() { /* onmouseover */
let cate1 = document.getElementById("cate1");
cate1.style.display = '';
}
function b(id) { /* onmouseout */
let cate = document.getElementById(id);
cate.style.display = 'none';
}
function c(idx) { // 대분류 항목에 마우스 올리면 중분류 나오게 하기
sel1 = idx;
let arr = cate2arr[idx]; // idx:0 [남성패션, 여성패션...]
let html = "";
for (i = 0; i < arr.length; i++) {
html += arr[i] + "<br/>";
}
let cate2 = document.getElementById("cate2");
cate2.innerHTML = html;
cate2.style.display = '';
}
function d() { // 중분류 배열 인덱스 초기화 (-1)
c(sel1);
}
</script>
</head>
<body>
<table style="border: 2px solid black">
<tr>
<td><img src="../img/cup_ca.png"
style="width: 100px; height: 100px" onmouseover="a()"
onmouseout="b('cate1')"></td>
<td colspan="3" style="font-size: 30pt; text-align: center">shop</td>
</tr>
<tr>
<td colspan="4"><img src="../img/1.jpg" id="img"
style="width: 600px; height: 300px"></td>
</tr>
<tr>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
</tr>
<tr>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
<td><a href="detail.html"><img src="../img/b.png"
style="width: 130px; height: 200px"></a></td>
</tr>
</table>
<div onmouseover="a()" onmouseout="b('cate1')" id="cate1"
style="display: none;">
<table style="width: 100px">
<tr onmouseover="c(0)" onmouseout="b('cate2')">
<td>의류</td>
</tr>
<tr onmouseover="c(1)" onmouseout="b('cate2')">
<td>가전</td>
</tr>
<tr onmouseover="c(2)" onmouseout="b('cate2')">
<td>가구</td>
</tr>
<tr onmouseover="c(3)" onmouseout="b('cate2')">
<td>잡화</td>
</tr>
</table>
</div>
<div onmouseover="d()" onmouseout="b('cate2')" id="cate2"
style="display: none;"></div>
</body>
</html>
제품상세화면
- 홈화면에서 하단의 상품 이미지를 누르면 제품상세 html 페이지로 넘어가기
- 이미지에 마우스를 올리면(onmouseover) 이미지 변하게 하기
- 주문 수량에 따라 (주문수량 * 가격) 값을 결제 금액에 띄우기 (onchange)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script>
function a(el){ /* 마우스 올리면 이미지 전환 */
let bigImg = document.getElementById("bigImg");
bigImg.src = el.src;
}
function b(){ /* 주문수량*가격 값을 결제금액에 띄우기 (onchange) */
let price = parseInt(document.getElementById("price").innerHTML);
let amount = parseInt(document.getElementById("amount").value);
document.getElementById("total").innerHTML = price*amount;
}
</script>
</head>
<body>
<h3>제품 상세 페이지</h3>
<table border="1" cellspacing='0'>
<tr>
<td>
<table>
<tr>
<td colspan="4"><img id="bigImg" src="../img/b.png"
style="width: 200px; height: 200px"></td>
</tr>
<tr>
<td><img src="../img/a.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
<td><img src="../img/b.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
<td><img src="../img/a.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
<td><img src="../img/b.png" style="width: 50px; height: 50px"
onmouseover="a(this)"></td>
</tr>
</table>
</td>
<td>
<table border="1" cellspacing='0'>
<tr><th>제품명</th><td>파란 하트</td></tr>
<tr><th>가격</th><td id="price">30000</td></tr>
<tr><th>주문수량</th><td><input type="number" id="amount" onchange="b()"></td></tr>
<tr><th>결제금액</th><td id="total"></td></tr>
<tr><th>결제</th><td><input type="button" value="바로결제">
<input type="button" value="장바구니"></td></tr>
</table>
</td>
</tr>
<tr><td colspan = "2"> 상품 상세 설명</td></tr>
<tr>
<td colspan = "2"> 봄이 생각나는 하트 <br/>
<img src="../img/b.png" style="width:400px; height:400px">
</td>
</table>
</body>
</html>